What is Spring Boot?
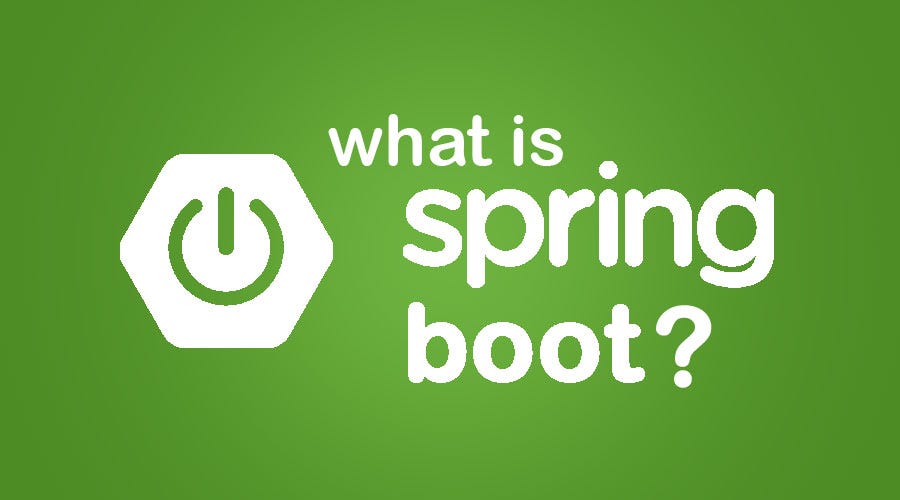
Spring Boot is an open-source Java-based framework used to create a microservice. It is developed by Pivotal Team and is used to build stand-alone and production-ready spring applications.
Spring Boot takes an opinionated view of the Spring platform and third-party libraries so you can get started with minimum fuss. Most Spring Boot applications need minimal Spring configuration.
Here are some of the benefits of using Spring Boot:
- Quick and easy to set up: Spring Boot makes it easy to create a new project and get it up and running quickly. You don’t need to spend time configuring Spring beans or other dependencies.
- Production-ready: Spring Boot applications are ready for production out of the box. They include features like embedded Tomcat or Jetty servers, automatic dependency management, and actuator endpoints for monitoring and management.
- Concise and readable code: Spring Boot applications are typically much more concise and readable than traditional Spring applications. This is because Spring Boot takes care of a lot of the boilerplate code for you.
- Community support: Spring Boot has a large and active community of users and developers. There are many resources available to help you get started with Spring Boot, including tutorials, documentation, and sample code.
What are the top use cases of Spring Boot?
Spring Boot is a popular framework for creating Java applications. It is used in a wide variety of industries and applications, but some of the top use cases include:
- Web applications: Spring Boot is a great choice for creating web applications. It provides a number of features that make it easy to build scalable and reliable web applications, such as embedded servers, automatic dependency management, and actuator endpoints for monitoring and management.
- Microservices: Spring Boot is also a popular choice for creating microservices. Microservices are small, independent services that communicate with each other over a network. Spring Boot makes it easy to create microservices that are easy to deploy, manage, and scale.
- APIs: Spring Boot is a great choice for creating APIs. APIs are used to expose functionality to other applications or systems. Spring Boot makes it easy to create APIs that are secure, scalable, and easy to use.
- Backend systems: Spring Boot is also a popular choice for creating backend systems. Backend systems are the systems that power the front end of an application. Spring Boot makes it easy to create backend systems that are reliable, scalable, and secure.
- Data science and machine learning: Spring Boot is also becoming a popular choice for data science and machine learning applications. Spring Boot makes it easy to build scalable and reliable applications that can process large amounts of data.
What are features of Spring Boot?
Spring Boot has a number of features that make it a great choice for creating Java applications. Here are some of the most important features:
- Autoconfiguration: Spring Boot automatically configures many of the Spring Framework’s features, such as dependency injection, servlets, and security. This saves you a lot of time and effort in configuring your application.
- Starter dependencies: Spring Boot provides starter dependencies for a variety of technologies, such as web development, database access, and messaging. This makes it easy to add these technologies to your application with just a few lines of code.
- Actuator endpoints: Spring Boot provides actuator endpoints that allow you to monitor and manage your application. These endpoints can be used to get information about your application’s health, metrics, and logs.
- Production-ready features: Spring Boot includes a number of features that make it production-ready, such as embedded servers, security, and logging. This means that you can deploy your application to production without having to do a lot of additional configuration.
- Concise and readable code: Spring Boot applications are typically much more concise and readable than traditional Spring applications. This is because Spring Boot takes care of a lot of the boilerplate code for you.
- Large and active community: Spring Boot has a large and active community of users and developers. There are many resources available to help you get started with Spring Boot, including tutorials, documentation, and sample code.
What is the workflow of Spring Boot?
The workflow of developing applications using Spring Boot follows a series of steps that encompass project setup, development, testing, and deployment.
The workflow of Spring Boot is as follows:
- Create a new Spring Boot project. You can do this using the Spring Boot Initializr website or the Spring Boot CLI.
- Add the dependencies you need. Spring Boot provides starter dependencies for a variety of technologies, such as web development, database access, and messaging. You can add these dependencies to your project using the Spring Boot Initializr or the Spring Boot CLI.
- Write your code. Your code will typically consist of controllers, services, and repositories. Controllers handle HTTP requests, services perform business logic, and repositories interact with the database.
- Configure your application. Spring Boot uses annotations to configure your application. You can use annotations to configure things like the port your application listens on, the database you use, and the security settings.
- Run your application. You can run your application using the Spring Boot Maven plugin or the Spring Boot Gradle plugin.
- Test your application. You can test your application using JUnit or the Spring Boot Test framework.
How Spring Boot Works & Architecture?
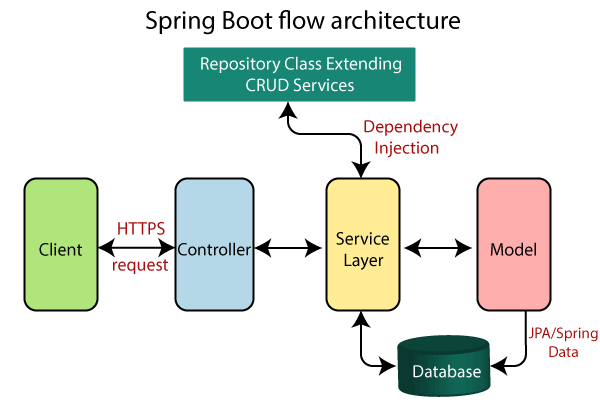
Spring Boot is a framework that helps you create Java applications quickly and easily. It is based on the Spring Framework, but it takes a more opinionated approach to configuration. This means that Spring Boot makes a lot of decisions for you, such as which dependencies to use and how to configure them. This can be a benefit, as it can save you time and effort. However, it can also be a drawback, as you may not have as much control over your application.
Spring Boot works by using a number of features, including:
- Autoconfiguration: Spring Boot automatically configures many of the Spring Framework’s features, such as dependency injection, servlets, and security. This saves you a lot of time and effort in configuring your application.
- Starter dependencies: Spring Boot provides starter dependencies for a variety of technologies, such as web development, database access, and messaging. This makes it easy to add these technologies to your application with just a few lines of code.
- Actuator endpoints: Spring Boot provides actuator endpoints that allow you to monitor and manage your application. These endpoints can be used to get information about your application’s health, metrics, and logs.
- Production-ready features: Spring Boot includes a number of features that make it production-ready, such as embedded servers, security, and logging. This means that you can deploy your application to production without having to do a lot of additional configuration.
How to Install and Configure Spring Boot?
Installing and configuring Spring Boot is a straightforward process. Spring Boot provides a variety of options for setting up and developing applications.
Here’s a step-by-step guide to get you started:
1. Prerequisites:
- Java Development Kit (JDK): Spring Boot requires JDK 8, 11, or 16 (depending on *the version you choose). You can download JDK from the official Oracle website or use OpenJDK.
- Integrated Development Environment (IDE): While not strictly required, using an IDE like IntelliJ IDEA or Eclipse can greatly enhance your development experience.
2. Install Spring Boot:
Spring Boot can be set up in multiple ways, but one of the most common methods is to use the Spring Initializr web interface or IDE integration:
Using Spring Initializr (Web Interface):
- Open your web browser and navigate to https://start.spring.io/.
- Choose the project settings, such as language (Java), Spring Boot version, packaging (JAR or WAR), and Java version.
- Select the dependencies you need for your project, such as “Spring Web” for web applications, “Spring Data JPA” for database access, and others.
- Click the “Generate” button to download a zip file containing your Spring Boot project.
Using IDE Integration:
Most modern IDEs provide integration with Spring Initializr, allowing you to create a Spring Boot project directly from within the IDE:
- Open your IDE (e.g., IntelliJ IDEA or Eclipse).
- Look for an option to create a new project. In IntelliJ IDEA, you can go to “File” > “New” > “Project” and select “Spring Initializr.”
- Choose project settings and dependencies similar to the steps mentioned above in the web interface approach.
- Follow the prompts to create the project.
3. Develop and Configure:
Once you’ve set up your Spring Boot project, you can start developing your application:
- Project Structure: Your Spring Boot project will have a standard directory structure, including source code (src/main/java), resources (src/main/resources), and tests (src/test).
- Application Class: Every Spring Boot application has a main class annotated with @SpringBootApplication. This class serves as the entry point for your application.
- Configuration: You can configure your application using properties files (application.properties or application.yml) located in the resources folder. These files can be used to customize various aspects of your application.
- Dependencies: Spring Boot’s dependencies are managed using build tools like Maven or Gradle. Your project’s dependencies are specified in the build configuration file (pom.xml for Maven or build.gradle for Gradle).
4. Run and Test:
You can run your Spring Boot application in various ways:
- IDE: If you’re using an IDE like IntelliJ IDEA or Eclipse, you can run the application by right-clicking the main class and selecting “Run” or “Debug.”
- Command Line: Navigate to your project’s root directory and use the following command to run the application:
./mvnw spring-boot:run # For Unix-like systems
mvnw.cmd spring-boot:run # For Windows
3. Packaging: To create an executable JAR file, you can use the following command:
./mvnw clean package
5. Access the Application:
By default, your Spring Boot application will run on port 8080. Open a web browser and navigate to http://localhost:8080 to access your application. If you’ve defined custom configurations, endpoints, or mappings, you can access those as well.
Fundamentals Tutorial of Spring Boot
Absolutely, here’s a step-by-step fundamentals tutorial of Spring Boot to help you get started:
1. Install Prerequisites:
- Java Development Kit (JDK) 8, 11, or 16.
- Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse (optional but recommended).
2. Create a Spring Boot Project:
You can use the Spring Initializr (https://start.spring.io/) to generate a Spring Boot project. Choose project settings, dependencies, and packaging. Download and extract the generated project.
3. Open Project in IDE:
Open the project in your chosen IDE. If you’re using IntelliJ IDEA, choose “Open” and select the project folder.
4. Explore Project Structure:
Take a look at the project structure. Key directories include:
- src/main/java: Java source code.
- src/main/resources: Configuration files and resources.
- src/test: Test-related code.
5. Create a Simple REST Controller:
Create a new class in the src/main/java directory. For example,
‘HelloController.java’:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, Spring Boot!";
}
}
6. Run the Application:
Run the application using your IDE or the command line:
./mvnw spring-boot:run # Unix-like systems
mvnw.cmd spring-boot:run # Windows
7. Access the Endpoint:
Open a web browser and navigate to http://localhost:8080/hello. You should see “Hello, Spring Boot!”.
8. Customize Configuration:
Create or modify the application.properties or application.yml file in the src/main/resources directory to customize configuration settings. For example, you can change the server port:
server.port=8081
9. Spring Boot Starters:
Explore Spring Boot starters to simplify dependency management. For example, you can use spring-boot-starter-web for web applications. Add these dependencies to your pom.xml or build.gradle.
10. Data Access (Optional):
Integrate databases using Spring Data JPA. Create entity classes, repositories, and services for database interactions.
11. Spring Boot Testing:
Write unit tests and integration tests for your application components. Use testing frameworks like JUnit and Mockito.
12. Spring Boot Actuator (Optional):
Integrate Spring Boot Actuator to expose endpoints for monitoring and managing your application.
13. Build and Package:
Build your application into a JAR or WAR file:
./mvnw clean package # Unix-like systems
mvnw.cmd clean package # Windows
14. Run the Packaged Application:
Run the JAR file you’ve built:
java -jar target/your-app-name.jar
15. Further Learning:
Explore more advanced features like Spring Security, data validation, handling exceptions, connecting to databases, creating RESTful APIs, using Thymeleaf for templates, and more.