How To display an “Incorrect email or password” message when the login response is unauthorized in Flutter
To display an “Incorrect email or password” message when the login response is unauthorized in Flutter, you can follow these steps:
Step: 1
Create a variable in your login page’s state to hold the error message. For example:
String errorMessage = '';
Step: 2
In your login function or login button’s onPressed callback, make the login request to the server using an HTTP client, such as http
or dio
. Handle the response to determine if it is unauthorized.
Step: 3
If the login response is unauthorized, update the error message variable with the appropriate error message. For example:
Future<http.Response> login(String email, String password) async {
Map data = {
"email": email,
"password": password,
};
var body = json.encode(data);
var url = Uri.parse(baseURL + 'login');
http.Response response = await http.post(
url,
headers: headers,
body: body,
);
print('OK! not hnfx');
print('OK! not');
if (response.statusCode == 200) {
var getData = json.decode(response.body);
_savetoken(getData["success"]["token"]);
_saveemail(email);
} else if (response.statusCode == null) {
// Display error message for incorrect email or password
errorSnackBar(context, 'Incorrect email or password');
}
return response;
}
Step: 4
In your UI, display the error message below the login form or wherever you want to show it. You can conditionally show the error message widget based on whether the error message is empty or not. For example:
class _LoginScreenState extends State<LoginScreen> {
String _email = '';
String _password = '';
AuthServices authServices = AuthServices();
set _errorMessage(String _errorMessage) {}
loginPress() async {
if (_email.isNotEmpty && _password.isNotEmpty) {
http.Response response = await authServices.login(_email, _password);
Map responseMap = json.decode(response.body);
print(response.statusCode);
if (response.statusCode == 200) {
final prefs = await SharedPreferences.getInstance();
final key = 'token';
final value = prefs.get(key) ?? 0;
if (value != 0) {
Navigator.push(
context,
MaterialPageRoute(
builder: (BuildContext context) => Dashboard(),
));
} else {
var getData = json.decode(response.body);
final prefs = await SharedPreferences.getInstance();
final key = 'token';
final value = getData["success"]["token"];
prefs.setString(key, value);
final pemail = 'email';
final pvalue = _email;
prefs.setString(pemail, pvalue);
if (value != 0) {
Navigator.push(
context,
MaterialPageRoute(
builder: (BuildContext context) => Dashboard(),
));
}
}
} else {
errorSnackBar(context, 'Incorrect email or password');
}
} else {
errorSnackBar(context, 'enter all required fields');
}
}
By following these steps, you can display the “Incorrect email or password” message when the login response is unauthorized in Flutter.
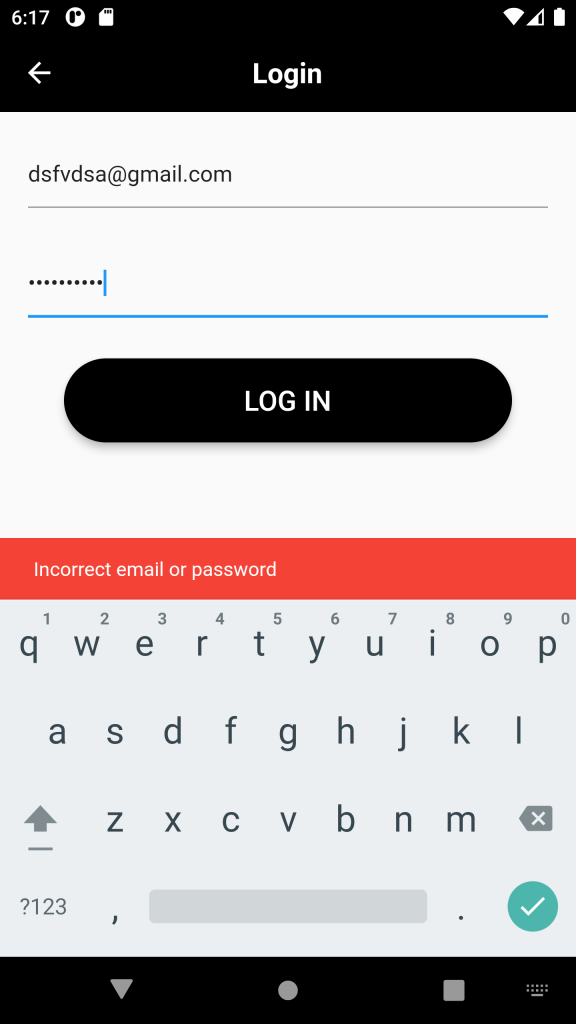