Fundamentals Tutorial of Vue.js
What is Vue.js?
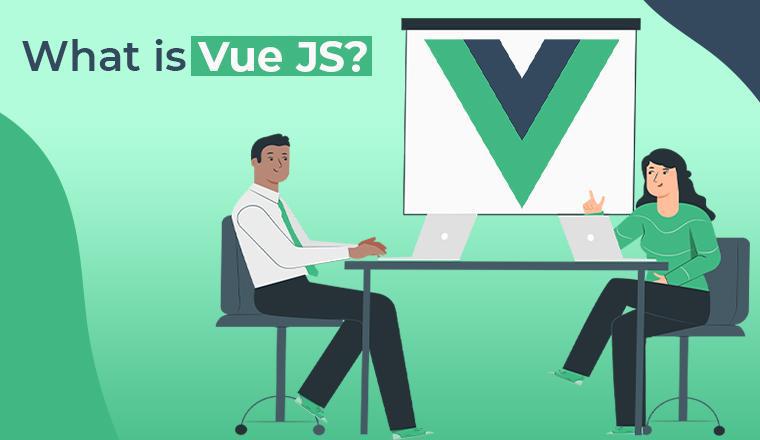
Vue.js (pronounced “view.js”) is a progressive JavaScript framework used for building user interfaces (UIs) and single-page applications (SPAs). It is designed to be flexible, approachable, and efficient, making it a popular choice among developers for creating interactive and dynamic web applications.
Here are some of the benefits of using Vue.js:
- Easy to learn: Vue.js is a relatively easy framework to learn, even for beginners. The documentation is clear and concise, and there are many tutorials and resources available online.
- Fast: Vue.js is a fast framework that can be used to create responsive and interactive user interfaces.
- Scalable: Vue.js is a scalable framework that can be used to build large and complex applications.
- Flexible: Vue.js is a flexible framework that can be used to build a variety of different types of user interfaces.
- Community: There is a large and active community of Vue.js developers who are always willing to help.
What are the top use cases of Vue.js?
Vue.js is a popular JavaScript framework for building user interfaces. It is used by a wide variety of companies, including Google, Netflix, and Alibaba. Here are some of the top use cases of Vue.js:
- Building single-page applications (SPAs): SPAs are web applications that load all of their content in a single page. This makes them more responsive and interactive than traditional web applications. Vue.js is a popular choice for building SPAs because it is easy to learn and use, and it is very performant.
- Building progressive web applications (PWAs): PWAs are web applications that can be installed on a user’s device and used like a native app. Vue.js is a good choice for building PWAs because it is easy to make Vue.js applications work offline and because it can be used to create hybrid apps that combine the features of web apps and native apps.
- Building custom user interfaces: Vue.js can be used to build custom user interfaces for a variety of applications, such as dashboards, e-commerce websites, and marketing websites. Vue.js is a good choice for building custom user interfaces because it is flexible and can be customized to meet the specific needs of an application.
- Building data-driven applications: Vue.js is a good choice for building data-driven applications because it is reactive and can automatically update the UI when the data changes. This makes it easy to build applications that are responsive and interactive.
- Building mobile applications: Vue.js can be used to build mobile applications using a variety of frameworks, such as Nuxt.js and Quasar. Vue.js is a good choice for building mobile applications because it is easy to learn and use, and it is very performant.
What are the features of Vue.js?
Vue.js is a JavaScript framework that is used to build user interfaces. It is a progressive framework, which means that it can be used incrementally. You can start with a simple component and then add more features as needed.
Vue.js has many features, but some of the most important ones include:
- Declarative: Vue.js uses a declarative programming style, which means that you describe what you want to do, not how to do it. This makes it easier to write code that is both readable and maintainable.
- Reactive: Vue.js is reactive, which means that it automatically updates the UI when the data changes. This makes it easy to build user interfaces that are responsive and interactive.
- Composition: Vue.js is based on the principle of composition, which means that you can build complex components by composing simpler components. This makes it easy to create reusable and maintainable code.
- Virtual DOM: Vue.js uses a virtual DOM, which means that it creates a copy of the DOM in memory and then updates the copy instead of the real DOM. This makes Vue.js very performant.
- Routing: Vue.js has a built-in routing system that makes it easy to navigate between different pages in your application.
- State management: Vue.js has a built-in state management system that makes it easy to manage the state of your application.
- Internationalization: Vue.js has built-in support for internationalization, which makes it easy to create applications that can be used by people all over the world.
What is the workflow of Vue.js?
The workflow of Vue.js can be divided into the following steps:
- Create a new project: The first step is to create a new project. You can do this using the Vue CLI, which is a command-line tool that can be used to create and manage Vue.js projects.
- Install the dependencies: The next step is to install the dependencies for your project. You can do this using the npm package manager.
- Create a component: The next step is to create a component. A component is a reusable piece of code that can be used to build your user interface.
- Register the component: Once you have created a component, you need to register it with Vue.js. This can be done by adding the component to the components property in your Vue instance.
- Use the component: Once you have registered the component, you can use it in your templates. To do this, you need to use the tag.
- Bind data to the component: You can bind data to the component by using the v-bind directive. The v-bind directive tells Vue.js to bind the data to the corresponding property in the component.
- Listen for events: You can listen for events from the component by using the von directive. The von directive tells Vue.js to listen to the event and execute the corresponding function when the event is triggered.
- Compile the code: Once you have finished writing your code, you need to compile it. This can be done using the Vue CLI.
- Run the application: Once the code has been compiled, you can run the application. You can do this by using the npm run serve command.
How Vue.js Works & Architecture?
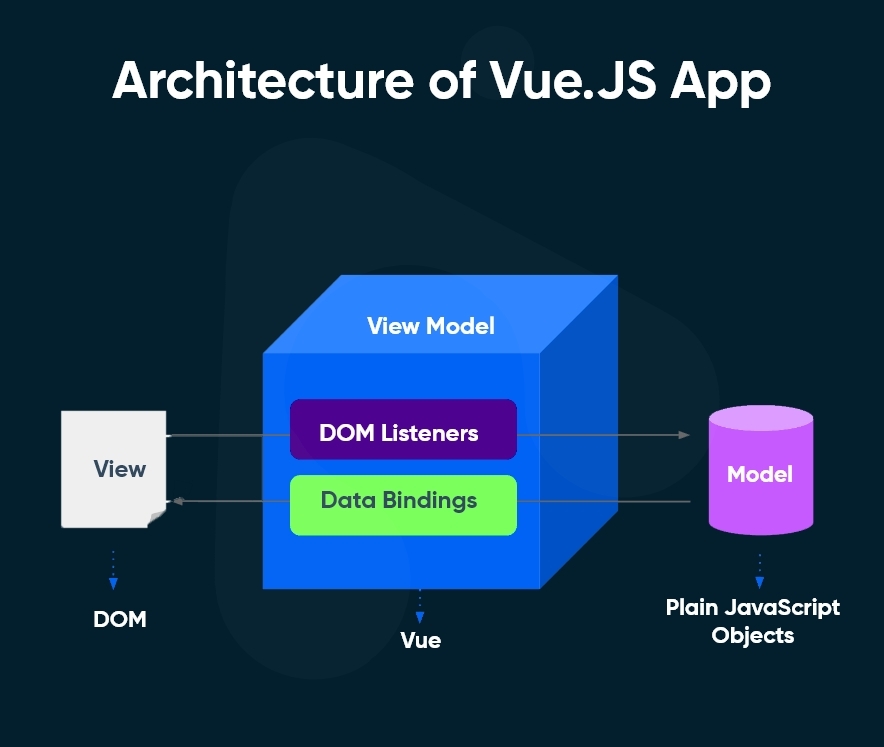
Vue.js is a progressive JavaScript framework for building user interfaces. It is a declarative framework, which means that you describe what you want to do, not how to do it. This makes it easier to write code that is both readable and maintainable.
Vue.js is based on the following principles:
- Declarative: Vue.js uses a declarative programming style, which means that you describe what you want to do, not how to do it. This makes it easier to write code that is both readable and maintainable.
- Reactive: Vue.js is reactive, which means that it automatically updates the UI when the data changes. This makes it easy to build user interfaces that are responsive and interactive.
- Composition: Vue.js is based on the principle of composition, which means that you can build complex components by composing simpler components. This makes it easy to create reusable and maintainable code.
How to Install and Configure Vue.js?
To install and configure Vue.js, you can follow these steps:
1. Installation:
You have a few options for installing Vue.js, but using Vue CLI is the most recommended and straightforward method.
Using Vue CLI (Command Line Interface):
Vue CLI is a command-line tool that helps you scaffold and manage Vue.js projects.
- First, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can download them from https://nodejs.org/.
- Open your terminal or command prompt.
- Install Vue CLI globally by running the following command:
npm install -g @vue/cli
- After the installation is complete, you can verify it by running:
vue --version
2. Create a New Vue Project:
- Navigate to the directory where you want to create your Vue project using the terminal.
- Create a new Vue project by running:
vue create project-name
- Follow the prompts to select configuration options for your project. You can choose default settings or manually configure features like additional tools, CSS preprocessing, and more.
3. Navigate to Project Directory:
- After the project is created, navigate to the project directory:
cd project-name
4. Run the Development Server:
- Start the development server to preview your Vue application in a web browser:
npm run serve
- This command compiles and serves your project. You’ll see a URL (usually http://localhost:8080/) in the terminal where you can access your app.
5. Configuration (Optional):
- Vue CLI provides a vue.config.js file in the project root for advanced configuration.
- This file can be used to modify various aspects of the build process, webpack configuration, and more.
6. Build for Production:
- When you’re ready to deploy your Vue.js application, you can build it for production using:
npm run build
- This command generates optimized and minified files in the dist directory that you can deploy to a web server.
Remember that Vue CLI handles most of the configuration for you, allowing you to focus on building your application. However, if you need to customize certain aspects of your project, the vue.config.js file is your go-to place.