Fundamentals Tutorial of NumPy
What is NumPy?
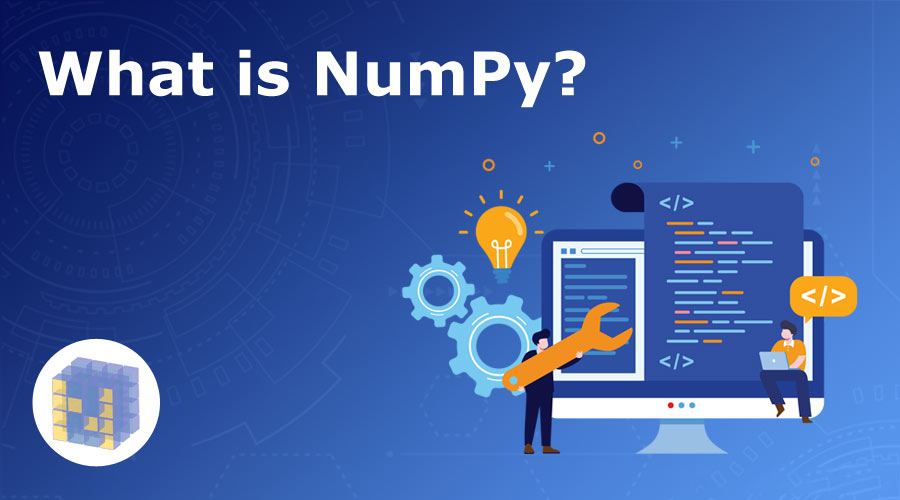
NumPy (Numerical Python) is a fundamental package in Python for numerical and scientific computing. It provides support for arrays, matrices, and a wide range of mathematical functions to work with these arrays efficiently. NumPy is a crucial building block for various data analysis, machine learning, and scientific computing tasks in Python.
What are the top use cases of NumPy?
NumPy is a powerful tool that can be used for a variety of tasks. If you are working with data in Python, I recommend learning NumPy.
Here are some additional details about the use cases of NumPy:
- Data analysis: NumPy can be used to load data from a variety of sources, such as CSV files, Excel spreadsheets, and databases. It can also be used to store data in memory for fast access. NumPy provides a number of functions for manipulating data, such as filtering, sorting, and aggregating. This makes it a powerful tool for data analysis tasks.
- Linear Algebra: NumPy provides a comprehensive library for linear algebra operations. This includes functions for matrix multiplication, matrix inversion, and eigenvalue decomposition. NumPy’s linear algebra functions are much faster than the equivalent functions in Python’s standard library. This makes NumPy a popular choice for tasks that require linear algebra, such as machine learning and computer graphics.
- Statistics: NumPy provides a number of statistical functions, such as mean, variance, and standard deviation. It also provides functions for hypothesis testing and fitting models to data. NumPy’s statistical functions are much faster than the equivalent functions in Python’s standard library. This makes NumPy a popular choice for statistical tasks.
- Machine learning: NumPy is a popular library for machine learning. It can be used to create and train machine learning models. NumPy’s multidimensional arrays are well-suited for storing and manipulating the large datasets that are often used in machine learning. NumPy’s vectorized operations can also be used to speed up the training of machine learning models.
What are the features of NumPy?
NumPy (Numerical Python) is a fundamental library in Python for numerical and scientific computing. It offers a wide range of features that make it a powerful tool for data manipulation, mathematical operations, and scientific computations.
NumPy is a powerful library for scientific computing in Python. It provides a number of features, including:
- N-dimensional arrays: NumPy arrays are multidimensional arrays that can store data of any type. This makes them well-suited for storing and manipulating large datasets.
- Vectorized operations: NumPy provides vectorized operations that can be used to perform operations on entire arrays at once. This can be much faster than performing the operations on individual elements of the array.
- Mathematical functions: NumPy provides a number of mathematical functions, such as sin, cos, and exp. This makes it a powerful tool for scientific computing tasks.
- Linear algebra functions: NumPy provides a comprehensive library for linear algebra operations. This includes functions for matrix multiplication, matrix inversion, and eigenvalue decomposition. This makes NumPy a popular choice for tasks that require linear algebra, such as machine learning and computer graphics.
- Statistical functions: NumPy provides a number of statistical functions, such as mean, variance, and standard deviation. This makes it a powerful tool for statistical tasks.
- Random number generation: NumPy provides a number of functions for generating random numbers. This makes it a powerful tool for Monte Carlo simulations and other tasks that require random numbers.
- Object-oriented programming support: NumPy supports object-oriented programming. This makes it a powerful tool for developing complex applications.
What is the workflow of NumPy?
The workflow of NumPy may seem daunting at first, but it is actually quite straightforward. Once you get the hang of it, you will be able to use NumPy to perform a variety of scientific computing tasks quickly and efficiently.
Here are some additional details about the workflow of NumPy:
- Importing NumPy: To import NumPy, you can use the following import statement:
import numpy as np
- Creating a NumPy array: To create a NumPy array, you can use the array() function. The array() function takes a list of elements as its argument and returns a NumPy array. For example, the following code creates a NumPy array with the numbers 1, 2, 3, and 4:
array = np.array([1, 2, 3, 4])
- Performing operations on a NumPy array: NumPy provides a variety of functions for performing operations on NumPy arrays. For example, the following code calculates the mean of the elements in the NumPy array:
mean = np.mean(array)
- Saving a NumPy array: To save a NumPy array to a file, you can use the save() function. The save() function takes the name of the file as its argument. For example, the following code saves the NumPy array to a file called my_array.npy
np.save('my_array.npy', array)
- Loading a NumPy array: To load a NumPy array from a file, you can use the load() function. The load() function takes the name of the file as its argument. For example, the following code loads the NumPy array from the file my_array.npy:
array = np.load('my_array.npy')
How NumPy Works & Architecture?
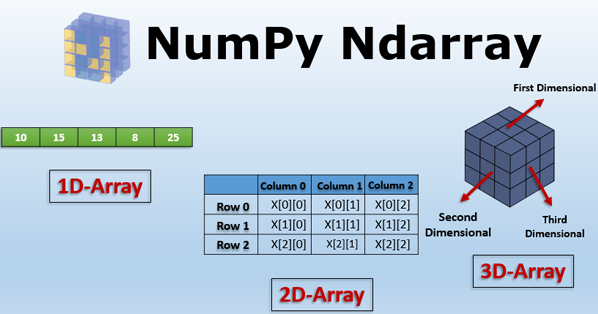
NumPy works by storing data in a contiguous block of memory. This makes it possible to perform operations on NumPy arrays much faster than on regular Python lists. NumPy arrays are also much more efficient for storing and manipulating large datasets.
The architecture of NumPy is relatively simple. It consists of a few core modules, including:
- The array module: The array module provides the core functionality for NumPy arrays. It includes functions for creating, manipulating, and accessing NumPy arrays.
- The math module: The math module provides a number of mathematical functions that can be used on NumPy arrays.
- The linear algebra module: The linear algebra module provides a comprehensive library for linear algebra operations on NumPy arrays.
- The random module: The random module provides functions for generating random numbers.
- The testing module: The testing module provides functions for testing NumPy arrays.
How to Install and Configure NumPy?
Installing and configuring NumPy is a fundamental step to start using its powerful numerical computing capabilities in Python. Here’s a step-by-step guide on how to install and configure NumPy:
Installing NumPy:
1. Install Python:
Ensure you have Python installed on your system. NumPy works with both Python 2 and Python 3, but it’s recommended to use Python 3 as Python 2 has reached its end of life.
2. Open a Command-Line Terminal:
On Windows, open the Command Prompt. On macOS and Linux, open the Terminal.
3. Install NumPy:
In the terminal, use the package manager pip (Python’s package installer) to install NumPy. Run the following command:
pip install numpy
This command will download and install the latest version of NumPy from the Python Package Index (PyPI).
Verifying Installation:
After installation, you can verify that NumPy is installed by opening a Python interpreter or a Jupyter Notebook and importing the library:
import numpy as np
# Create a simple array to test if NumPy is working
arr = np.array([1, 2, 3])
print(arr)
If you see the array printed without any errors, NumPy is successfully installed.
Configuring NumPy (Optional):
NumPy doesn’t require explicit configuration; it’s ready to use after installation. However, you can adjust its behavior by using the built-in configuration options and environment variables. Some useful configuration options include:
- Changing the display format for floating-point numbers (np.set_printoptions()).
- Adjusting the behavior of broadcasting rules and error handling.
- Controlling how NumPy handles NaN (Not-a-Number) values.
- Specifying the random number generator’s seed for reproducibility.