Fundamentals Tutorial of Function
What is Function?
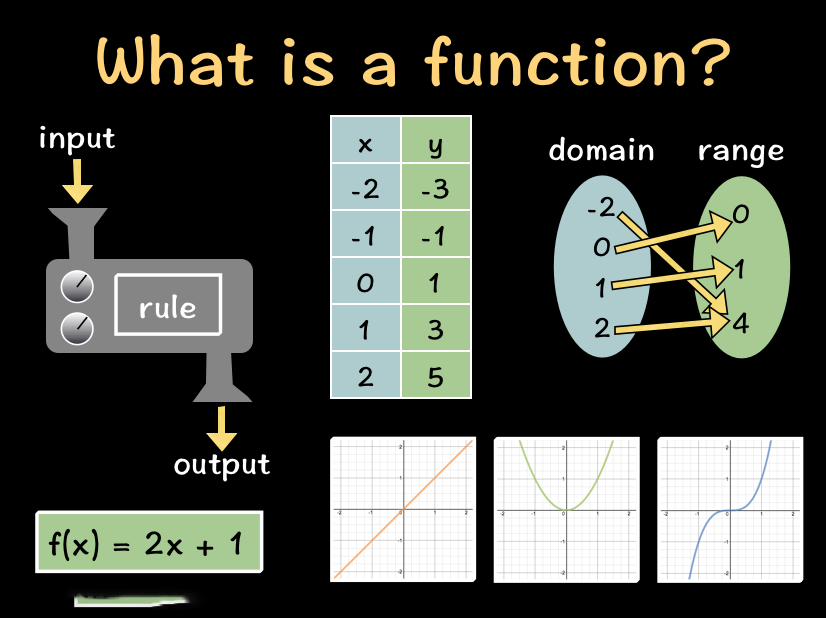
A function is a self-contained block of code that performs a specific task or operation. It takes one or more input values (arguments), processes them, and produces an output value. Functions play a crucial role in programming by promoting code reuse, modularity, and organization.
The syntax for defining a function in Scala is as follows:
def functionName(parameter1: DataType, parameter2: DataType): DataType = {
// Body of the function
return value
}
The def keyword is used to define a function. The functionName is the name of the function. The parameter1 and parameter2 are the parameters of the function. The DataType is the data type of the parameters. The return keyword is used to return a value from the function.
Functions can be called by using their name and passing the parameters. For example, the following code calls the factorial function with the parameter 5:
val result = factorial(5)
println(result)
This code will print the following output:
120
Functions can also be nested. This means that a function can be defined inside another function. For example, the following code defines a function nestedFactorial that calls the factorial function:
def nestedFactorial(n: Int): Int = {
def factorial(n: Int): Int = {
if (n == 0) {
return 1
} else {
return n * factorial(n - 1)
}
}
return factorial(n)
}
This code will print the same output as the previous code.
What are the top use cases of Function?
Here are some of the top use cases of functions in Scala:
- Calculations: Functions can be used to perform calculations, such as calculating the factorial of a number or the square root of a number.
- Data processing: Functions can be used to process data, such as sorting a list of numbers or finding the average of a set of numbers.
- Input/output: Functions can be used to read data from input sources, such as the console or a file, and to write data to output sources, such as the console or a file.
- Control flow: Functions can be used to control the flow of execution of a program, such as by checking if a condition is true or false or by looping through a sequence of statements.
- Error handling: Functions can be used to handle errors, such as by printing an error message or by terminating the program.
- Testing: Functions can be used to test other functions, such as by providing test cases that check the functionality of the function.
What are the features of Function?
Functions are a core feature of programming languages that enable developers to encapsulate and organize code for reusability and modularity. Depending on the programming language, functions may have various features and capabilities.
Here are some of the most important features of functions in Scala:
- Parameter passing: Functions can take parameters, which are values that are passed to the function when it is called. The parameters can be of any type, including primitive types, classes, and objects.
- Return values: Functions can return a value, which is a value that is produced by the function and can be used by the caller of the function. The return value can be of any type, including primitive types, classes, and objects.
- Recursion: Functions can be recursive, which means that they can call themselves. This can be useful for solving problems that involve repeated subproblems.
- Higher-order functions: Functions can take functions as parameters or return functions as values. This makes it possible to write code that is more modular and reusable.
- Currying: Currying is a technique for transforming a function that takes multiple parameters into a function that takes a single parameter. This can be useful for making code more concise and readable.
What is the workflow of Function?
Functions in Scala have a three-step workflow:
- Definition: The function is defined using the def keyword. The definition includes the name of the function, the parameters of the function, and the body of the function.
- Call: The function is called by using its name and passing the parameters. The parameters are the values that are passed to the function when it is called.
- Return: The function returns a value, which is the value that is produced by the function and can be used by the caller of the function.
How Function Works & Architecture?
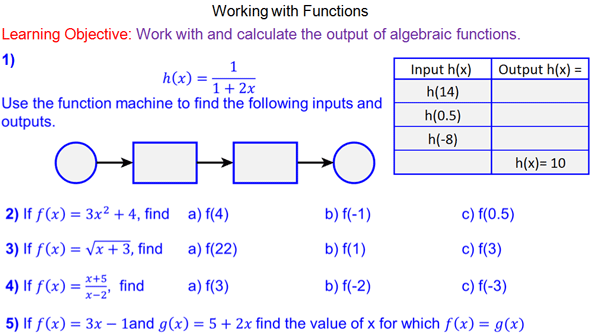
Functions in Scala work by first defining the function using the def keyword. The definition includes the name of the function, the parameters of the function, and the body of the function. The name of the function is a unique identifier for the function. The parameters of the function are the values that are passed to the function when it is called. The body of the function is the code that is executed when the function is called.
Once the function is defined, it can be called by using its name and passing the parameters. The parameters are the values that are passed to the function when it is called. The function can be called from anywhere in the code. When a function is called, the Scala compiler first checks to see if the function has been defined. If the function has been defined, the compiler then executes the body of the function. The body of the function is executed once for each time the function is called. The return value of the function is the value that is produced by the function and can be used by the caller of the function. The return value of the function can be of any type, including primitive types, classes, and objects.
The architecture of functions in Scala is based on the concept of closures. A closure is a function that has access to the values of the variables that were in scope when the function was defined. This allows functions in Scala to be more powerful and versatile than functions in other programming languages.
Here are some of the key features of the architecture of functions in Scala:
- Functions are first-class citizens: Functions in Scala are first-class citizens, which means that they can be treated like any other value. This means that functions can be passed as parameters to other functions, returned from functions, and stored in variables.
- Functions are closures: Functions in Scala are closures, which means that they have access to the values of the variables that were in scope when the function was defined. This allows functions in Scala to be more powerful and versatile than functions in other programming languages.
- Functions are lazy: Functions in Scala are lazy, which means that they are not evaluated until they are actually needed. This can improve the performance of Scala code by avoiding the unnecessary evaluation of functions.
How to Install and Configure Function?
Here are the steps on how to install and configure functions in Scala:
- Install the Scala compiler: You can download the Scala compiler from the Scala website. Once you have downloaded the Scala compiler, you need to extract the archive to a directory of your choice.
- Configure the Scala environment: You need to configure the Scala environment so that your computer knows where to find the Scala compiler. You can do this by adding the following line to your ~/.bashrc file:
export SCALA_HOME=<path to scala installation>
Replace <path to scala installation> with the path to the directory where you extracted the Scala compiler.
3. Verify that Scala is installed correctly: You can verify that Scala is installed correctly by running the following command in your terminal:
scala -version
This command should output the version of Scala that is installed on your computer.
4. Install an IDE: There are a number of IDEs that you can use to develop Scala applications. Some popular IDEs for Scala include IntelliJ IDEA, Eclipse, and Visual Studio Code.
5. Create a Scala project: Once you have installed an IDE, you can create a Scala project. The steps for creating a Scala project will vary depending on the IDE that you are using.
6. Write some Scala code: Once you have created a Scala project, you can start writing some Scala code. Scala code is typically written in text files with the .scala extension.
7. Compile and run your Scala code: Once you have written some Scala code, you need to compile and run it. You can do this by running the following command in your terminal:
scalac <path to scala file>
This command will compile the Scala file and create a bytecode file. You can then run the bytecode file by running the following command:
scala <path to bytecode file>
This command will run the Scala code and produce the desired output.
8. Test your Scala code: Once you have written and compiled your Scala code, you need to test it. You can do this by using a testing framework such as ScalaTest or Specs2.
9. Deploy your Scala code: Once you have tested your Scala code and it is working correctly, you can deploy it to production. You can deploy Scala code to a variety of different environments, including servers, cloud platforms, and mobile devices.