Fundamental Tutorials of TypeScript
What is TypeScript?
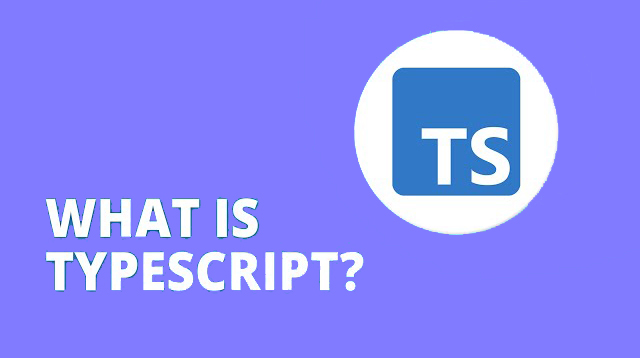
TypeScript is a typed superset of JavaScript that adds optional static typing to the language. TypeScript is designed to be a safer and more reliable way to write JavaScript code.
Here are some of the benefits of using TypeScript:
- Static typing: TypeScript adds static typing to JavaScript, which means that the compiler can check the types of variables and expressions at compile time. This can help to catch errors earlier in the development process and prevent runtime errors.
- Code completion: TypeScript’s type system makes it easier to use code completion in your IDE. This can help you to write code more quickly and accurately.
- Refactoring: TypeScript’s type system makes it easier to refactor your code. This is because the compiler can automatically infer the types of variables and expressions, so you don’t have to worry about changing the types manually.
- Interoperability: TypeScript is fully interoperable with JavaScript. This means that you can use TypeScript code in JavaScript projects and vice versa.
What are the top use cases of TypeScript?
TypeScript is a versatile language that can be used for a variety of purposes. Here are some of the top use cases of TypeScript:
- Web development: TypeScript is a popular choice for developing web applications. It can be used to create single-page applications, server-side applications, and progressive web apps.
- Mobile development: TypeScript can also be used for mobile development. It can be used to create native iOS and Android applications.
- Server-side development: TypeScript can be used for server-side development. It can be used to create APIs, microservices, and web servers.
- Desktop development: TypeScript can also be used for desktop development. It can be used to create desktop applications for Windows, macOS, and Linux.
- Game development: TypeScript can be used for game development. It can be used to create 2D and 3D games.
What are the features of TypeScript?
TypeScript is a typed superset of JavaScript that adds optional static typing to the language. TypeScript is designed to be a safer and more reliable way to write JavaScript code.
Here are some of the features of TypeScript:
- Static typing: TypeScript adds static typing to JavaScript, which means that the compiler can check the types of variables and expressions at compile time. This can help to catch errors earlier in the development process and prevent runtime errors.
- Interfaces: TypeScript interfaces allow you to define the shape of a type. This can be useful for ensuring that your code is consistent and for making your code more readable.
- Classes: TypeScript classes are similar to JavaScript classes, but they offer additional features, such as inheritance and decorators.
- Generics: TypeScript generics allow you to create functions and classes that can work with different types of data. This can be useful for making your code more reusable and flexible.
- Modules: TypeScript modules allow you to organize your code into logical units. This can help to make your code easier to understand and maintain.
- Decorators: TypeScript decorators allow you to modify the behavior of your code at runtime. This can be useful for adding functionality to your code or for changing the way that your code is executed.
What is the workflow of TypeScript?
The workflow of TypeScript is as follows:
- Write TypeScript code: You can write TypeScript code in any text editor. TypeScript code looks similar to JavaScript code, but it has some additional features, such as static typing and interfaces.
- Compile TypeScript code: Once you have written your TypeScript code, you need to compile it into JavaScript code. This can be done using the TypeScript compiler, which is included with the TypeScript installation.
- Run the JavaScript code: Once your TypeScript code has been compiled into JavaScript code, you can run it in a web browser or in a Node.js environment.
How TypeScript Works & Architecture?
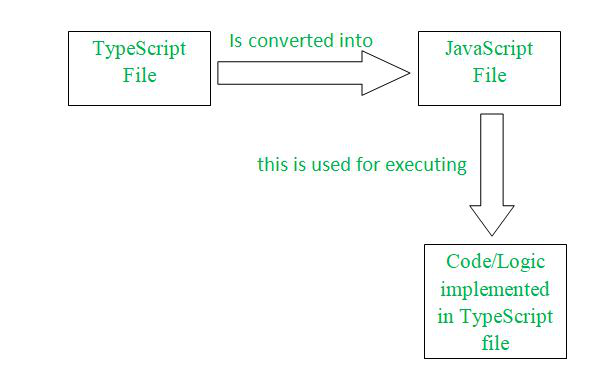
TypeScript works by first compiling your TypeScript code into JavaScript code. The TypeScript compiler is responsible for checking the types of your variables and expressions and generating JavaScript code that is equivalent to your TypeScript code. The TypeScript compiler is also responsible for generating type annotations for your JavaScript code. These type annotations can be used by TypeScript IDEs to provide code completion and other features. Once your TypeScript code has been compiled into JavaScript code, it can be run in a web browser or in a Node.js environment.
The architecture of TypeScript is relatively simple. It consists of the following components:
- The TypeScript compiler: The TypeScript compiler is responsible for checking the types of your variables and expressions and generating JavaScript code that is equivalent to your TypeScript code.
- The TypeScript type system: The TypeScript type system is used to define the types of variables and expressions in TypeScript code.
- The TypeScript language service: The TypeScript language service is responsible for providing code completion and other features to TypeScript IDEs.
- The TypeScript runtime: The TypeScript runtime is responsible for executing TypeScript code that has been compiled into JavaScript code.
How to Install and Configure TypeScript?
Here are the steps on how to install and configure TypeScript:
1. Install TypeScript: You can install TypeScript using a package manager, such as npm or yarn.
npm install -g typescript
or
yarn global add typescript
This will install the TypeScript compiler and the TypeScript language service globally on your machine.
2. Set up a TypeScript project: If you are creating a new TypeScript project, you need to create a tsconfig.json file. This file tells the TypeScript compiler how to compile your code.
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"outDir": "./dist"
}
}
The tsconfig.json file in this example tells the TypeScript compiler to compile your code to ES5, use the CommonJS module system, and output the compiled code to the dist folder.
3. Use a TypeScript IDE: There are a number of TypeScript IDEs available, such as Visual Studio Code, WebStorm, and Atom. These IDEs can help you to write and debug TypeScript code more easily.
[…] Fundamental Tutorials of TypeScript […]