Fundamental Tutorials of Swift
What is Swift?
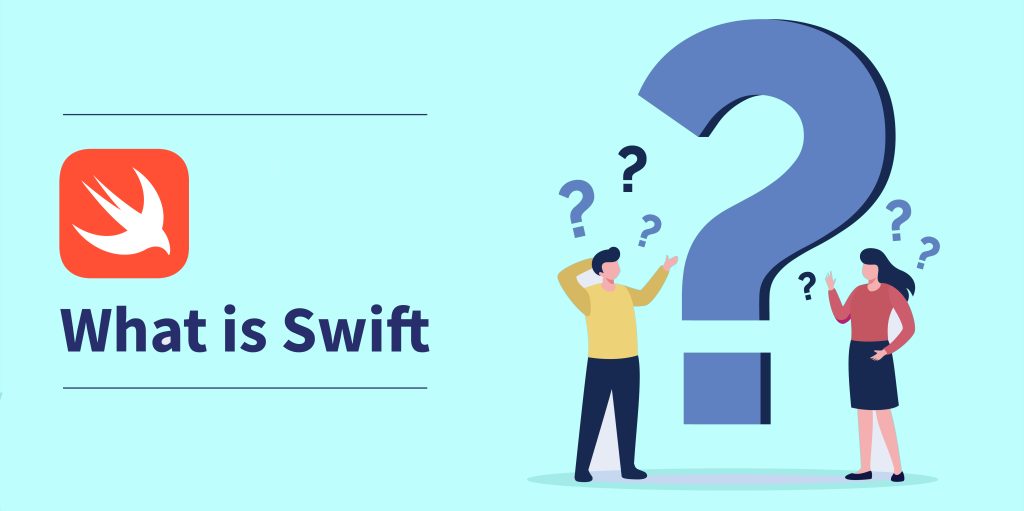
Swift is a general-purpose, multi-paradigm, compiled programming language developed by Apple Inc. and the open-source community. It was first released in 2014 as a replacement for Apple’s earlier programming language, Objective-C. Swift is designed to be safer, more modern, and more expressive than Objective-C.
Here are some of the key features of Swift:
- Safe by design: Swift has a number of features that help to prevent common programming errors, such as memory leaks and undefined behavior.
- Modern: Swift incorporates many modern programming language features, such as generics, closures, and optional.
- Expressive: Swift has a concise and expressive syntax that makes it easy to read and write code.
- Fast: Swift code is compiled into native code, which makes it fast and efficient.
- Extensible: Swift is an open-source language, which means that anyone can contribute to its development.
What is top use cases of Swift?
Swift is a versatile language that can be used for a variety of tasks.
Here are some of the top use cases of Swift:
- Building iOS, iPadOS, macOS, tvOS, and watchOS apps: Swift is the official language for developing apps for Apple’s platforms. It’s a great choice for building native apps that are fast, efficient, and easy to use.
- Creating server-side applications: Swift can also be used to create server-side applications. It’s a great choice for building scalable and reliable applications that can handle a lot of traffic.
- Writing command-line tools: Swift is a great choice for writing command-line tools. Its concise syntax and powerful features make it easy to write efficient and effective tools.
- Machine learning and artificial intelligence: Swift is a good choice for developing machine learning and artificial intelligence applications. It’s fast, expressive, and easy to use, making it a good fit for complex and demanding applications.
- Web development: Swift can also be used for web development. It’s not as popular as other languages for web development, but it’s a good choice for building high-performance and secure web applications.
What are feature of Swift?
Swift is a powerful and expressive programming language with a number of features that make it a great choice for a variety of tasks.
Here are some of the key features of Swift:
- Safe by design: Swift has a number of features that help to prevent common programming errors, such as memory leaks and undefined behavior. For example, Swift uses automatic memory management to free up memory when it is no longer needed. This helps to prevent memory leaks, which can cause programs to crash.
- Modern: Swift incorporates many modern programming language features, such as generics, closures, and optional. These features make Swift a powerful and flexible language that can be used to solve a variety of problems.
- Expressive: Swift has a concise and expressive syntax that makes it easy to read and write code. For example, Swift uses type inference to automatically determine the type of a variable or expression. This helps to make code more readable and less error-prone.
- Fast: Swift code is compiled into native code, which makes it fast and efficient. This is important for applications that need to perform well, such as games and graphics-intensive apps.
- Extensible: Swift is an open-source language, which means that anyone can contribute to its development. This makes Swift a vibrant and growing language with a large community of developers.
What is the workflow of Swift?
The workflow of Swift is the process of developing a Swift application. It can be broken down into the following steps:
- Planning: The first step is to plan your application. This includes defining the scope of the application, identifying the features that you want to include, and creating a timeline for development.
- Design: Once you have a plan, you can start designing your application. This includes creating wireframes and mockups of the user interface and defining the data structures and algorithms that you will use.
- Coding: The next step is to start coding your application. This is where you will put all of your plans and designs into code.
- Testing: Once you have some code, you need to start testing it. This will help you to identify and fix bugs before you release your application to the public.
- Deployment: Once your application is tested and ready to go, you can deploy it to the App Store or another platform.
- Maintenance: Once your application is deployed, you need to maintain it. This includes fixing bugs, adding new features, and updating the application for new platforms or devices.
How Swift Works & Architecture?
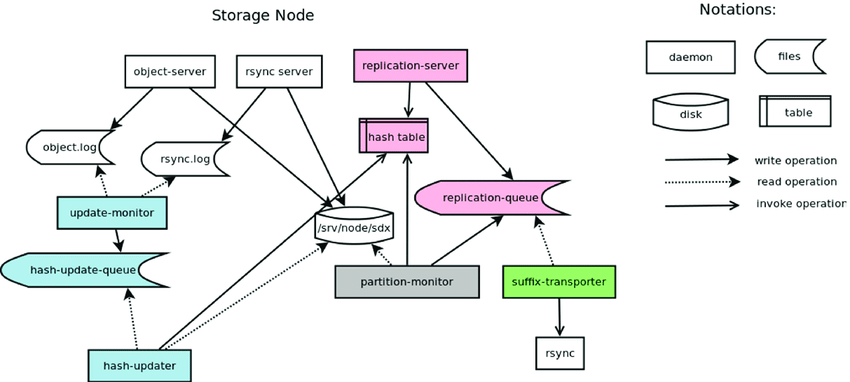
Swift is a compiled language, which means that it is converted into machine code before it is executed. This makes Swift fast and efficient. Swift code is compiled using the LLVM compiler infrastructure. Swift is a statically typed language, which means that the types of variables and expressions are known at compile time. This helps to prevent errors and makes code more reliable. Swift also supports type inference, which allows the compiler to automatically determine the type of a variable or expression based on its value.
Swift is a garbage-collected language, which means that the compiler automatically manages memory allocation and deallocation. This makes Swift easier to use and prevents memory leaks. Swift is a modular language, which means that it is made up of a collection of modules. Modules can be imported into other modules, which makes it easy to reuse code. Swift is an open-source language, which means that it is free to use and modify. This makes Swift a vibrant and growing language with a large community of developers.
The architecture of Swift is based on the following principles:
- Safety: Swift is designed to be safe, with features such as type safety and memory safety that help to prevent errors.
- Performance: Swift is designed to be fast and efficient, with features such as automatic memory management and garbage collection.
- Expressiveness: Swift is designed to be expressive, with a concise and easy-to-read syntax.
- Modularity: Swift is designed to be modular, with modules that can be imported into other modules.
- Open-source: Swift is designed to be open-source, with a large community of developers that can contribute to its development.
How to Install and Configure Swift?
Installing and configuring Swift depends on your target platform (macOS, Linux, or other supported platforms). Below are the steps to install and configure Swift on macOS and Ubuntu Linux, which are two commonly used platforms for Swift development:
1. Installing Swift on macOS:
Swift comes bundled with Xcode, Apple’s integrated development environment. Here’s how you can install Swift on macOS:
Install Xcode:
- Open the App Store on your macOS device.
- Search for “Xcode” and install it. Xcode includes the Swift compiler and development tools.
Verify Swift Installation:
- Open Terminal (you can find it in Applications > Utilities).
- Type swift –version and press Enter.
- You should see information about the installed Swift version if Xcode is successfully installed.
2. Installing Swift on Ubuntu Linux:
To install Swift on Ubuntu Linux, you’ll need to download the Swift binaries from the official website:
Add Swift Binaries Repository:
- Open Terminal.
- Run the following commands to add the Swift repository and key:
sudo apt update
sudo apt install clang libicu-dev
wget -q -O - https://swift.org/keys/all-keys.asc | sudo gpg --import -
sudo wget -q -O - https://swift.org/keys/swift-signing.gpg | sudo gpg --import -
Download and Install Swift:
- Determine the version you want to install by visiting the Swift download page.
- In Terminal, replace VERSION with the Swift version you want to install and run the following commands:
SWIFT_VERSION=VERSION
wget "https://swift.org/builds/swift-$SWIFT_VERSION-release/ubuntu2004/swift-$SWIFT_VERSION-RELEASE/swift-$SWIFT_VERSION-RELEASE-ubuntu20.04.tar.gz"
tar xzf "swift-$SWIFT_VERSION-RELEASE-ubuntu20.04.tar.gz"
sudo mv "swift-$SWIFT_VERSION-RELEASE-ubuntu20.04" /usr/share/swift
export PATH=/usr/share/swift/usr/bin:"${PATH}"
Verify Swift Installation:
- Close and reopen the Terminal to update the environment variables.
- Run Swift –version to verify that Swift is correctly installed.
3. Configure Swift:
After installing Swift, you may want to configure your development environment:
Text Editor/IDE:
- You can use Xcode for macOS development.
- For Linux, you can use a text editor like Visual Studio Code or an IDE like JetBrains CLion with Swift plugin.
Version Control:
- Consider using Git for version control to manage your Swift projects.
Package Manager:
- Swift has a package manager called Swift Package Manager (SPM) that helps manage dependencies and project structure.
- You can initialize a new Swift Package Manager project using the Swift package init command.
[…] Fundamental Tutorials of Swift […]