Fundamental Tutorials of String
What is String?
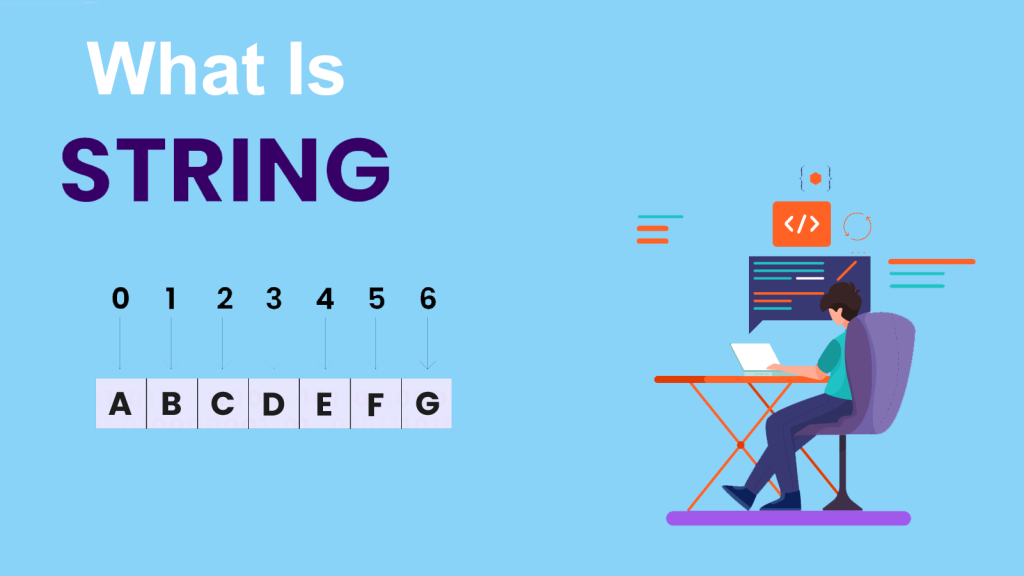
A string is a sequence of characters, typically used to represent text in computer programming. Characters within a string can include letters, numbers, symbols, and whitespace. Strings are a fundamental data type in most programming languages, and they play a crucial role in handling and manipulating textual data.
In programming, strings are used for a variety of purposes:
1. Text Representation: Strings are the primary way to represent and manipulate text data, such as names, addresses, descriptions, and more.
2. Input and Output: Strings are often used to capture user input from keyboards or other input devices and to display information as output on screens or in files.
3. Data Processing: Strings can be processed and manipulated using various operations, such as concatenation (joining strings), splitting (breaking strings into parts), and searching (finding substrings within strings).
4. Formatting: Strings can be formatted to achieve desired display patterns, such as adding placeholders for dynamic values or controlling capitalization.
5. Serialization: Strings are used to convert complex data structures (objects, arrays, etc.) into a format that can be stored or transmitted, such as JSON or XML.
6. Regular Expressions: Strings are often used with regular expressions (regex) to perform pattern matching and manipulation tasks.
7. URLs and Paths: Strings are used to represent URLs, file paths, and directory structures.
8. Error Messages and Logging: Strings are commonly used to display error messages to users or log information for debugging purposes.
In many programming languages, strings are usually enclosed in quotation marks (single or double) to indicate that they are a sequence of characters. For example:
- In Python: “Hello, World!”
- In Java: “Welcome to Programming”
- In JavaScript: ‘This is a string’
What is top use cases of String?
Strings are used in a variety of ways in programming. Here are some of the top use cases of strings:
- Storing text: Strings are often used to store text data. For example, you could use a string to store the name of a user, the contents of a file, or the output of a calculation.
- Formatting output: Strings can be used to format output in a variety of ways. For example, you could use a string to format the output of a calculation to two decimal places or to insert a variable into a string.
- Validating input: Strings can be used to validate input from users. For example, you could use a string to check if a user entered a valid email address or phone number.
- Searching and manipulating text: Strings can be used to search and manipulate text. For example, you could use a string to find all of the occurrences of a certain word in a text document or to replace all of the occurrences of a certain word with another word.
- Cryptography: Strings can be used in cryptography to encrypt and decrypt data. For example, you could use a string to encrypt a password or decrypt a message that was sent to you.
What are feature of String?
Here are some of the features of strings:
- Immutability: Strings are immutable, which means that they cannot be changed once they are created. This is different from other data types, such as lists, which can be changed.
- Indexing: Strings can be indexed, which means that you can access individual characters in a string using their position. For example, the character at position 0 in the string “Hello, world!” is the letter “H”.
- Slicing: Strings can be sliced, which means that you can extract substrings from a string. For example, the substring “Hello” can be extracted from the string “Hello, world!” using the slice [0:5].
- Concatenation: Strings can be concatenated, which means that you can combine two strings together. For example, the string “Hello, world!” can be concatenated with the string “Goodbye!” to create the string “Hello, world! Goodbye!”.
- Formatting: Strings can be formatted, which means that you can insert variables into a string. For example, the string “Hello, {name}!” can be formatted with the variable {name} to create the string “Hello, World!”.
- Regular expressions: Regular expressions are a powerful tool for searching and manipulating strings. Regular expressions can be used to find specific patterns in strings, such as all of the occurrences of a certain word or all of the phone numbers in a text document.
What is the workflow of String?
The workflow of string is the process of creating, manipulating, and using strings in programming. Here are the basic steps involved in the workflow of strings:
- Create a string: Strings can be created in a variety of ways. For example, you can create a string by enclosing a sequence of characters in single quotes (‘) or double quotes (“).
- Manipulate a string: Strings can be manipulated in a variety of ways. For example, you can slice strings to extract substrings, concatenate strings to combine them together, and format strings to insert variables.
- Use a string: Strings can be used in a variety of ways in programming. For example, you could use a string to store text data, to format output, to validate input, or to search and manipulate text.
How String Works & Architecture?
A string is a sequence of characters. It is a data type that is used to store text data. Strings can be enclosed in single quotes (‘) or double quotes (“).
In Python, strings are immutable, which means that they cannot be changed once they are created. However, you can create a new string that is a copy of an existing string.
Strings can be manipulated in a variety of ways. For example, you can slice strings to extract substrings, concatenate strings to combine them together, and format strings to insert variables.
Here is how strings work in Python:
- Strings are stored as a sequence of characters in memory. The characters are stored as integers, which represent their ASCII code values.
- Strings are immutable, which means that they cannot be changed once they are created. This means that you cannot change the characters in a string, or the order of the characters in a string.
- Strings can be manipulated using a variety of methods. These methods allow you to extract substrings from a string, combine strings together, and format strings.
How to Install and Configure String?
You don’t need to install strings in Python. Strings are a built-in data type in Python, so they are always available.
To configure strings, you can use the following methods:
The string.format() method: The string.format() method allows you to insert variables into a string. For example, the following code uses the string.format() method to insert the variable name into the string “Hello, {name}!”:
Python
name = "World"
str = "Hello, {name}!".format(name=name)
print(str)
The string.replace() method: The string.replace() method allows you to replace all occurrences of a certain substring with another substring. For example, the following code uses the string.replace() method to replace all occurrences of the letter “e” in the string “Hello, world!” with the letter “a”:
Python
str = "Hello, world!"
str = str.replace("e", "a")
print(str)
The string.split() method: The string.split() method allows you to split a string into a list of substrings. For example, the following code uses the string.split() method to split the string “Hello, world!” into a list of substrings:
Python
str = "Hello, world!"
substrings = str.split(",")
print(substrings)
These are just a few of the methods that you can use to configure strings in Python. For more information, you can refer to the Python documentation on strings.
[…] Fundamental Tutorials of String […]