Fundamental Tutorials of JSON
What is JSON?
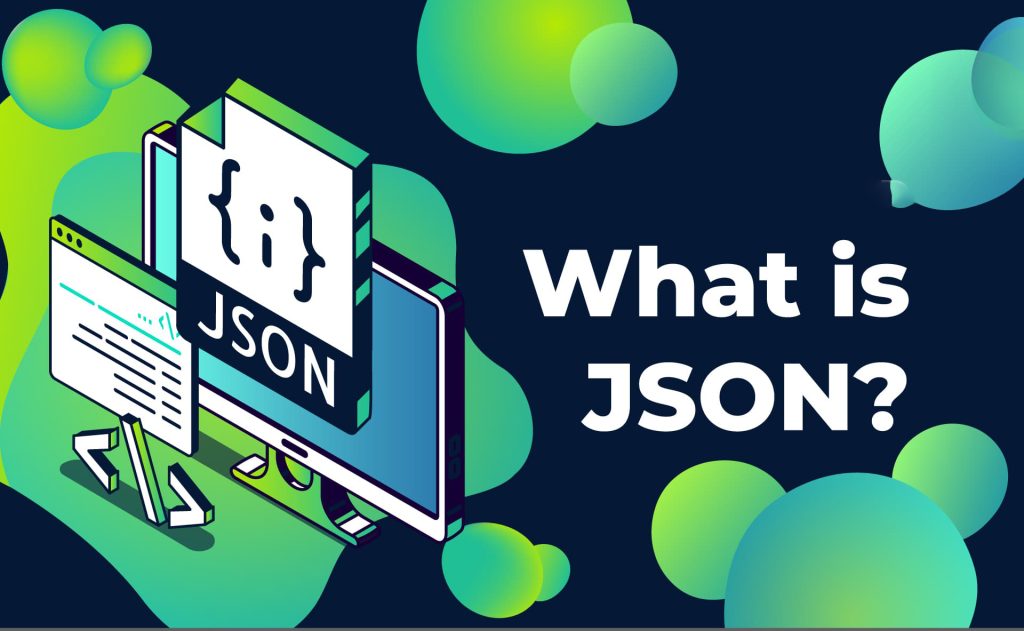
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and for machines to parse and generate. It is often used to transmit data between a server and a web application and store configuration and data in various programming environments. JSON is language-independent, making it a popular choice for exchanging data between different programming languages.
JSON is designed to be simple and concise, with its syntax primarily derived from the JavaScript object literal notation. It consists of key-value pairs, where keys are strings enclosed in double quotes, and values can be strings, numbers, boolean values, objects, arrays, or null. JSON values cannot include functions or undefined values.
Here is an example of JSON:
JSON
{
"name": "John Doe",
"age": 30,
"hobbies": ["coding", "reading", "hiking"]
}
What are the top use cases of JSON?
JSON is a popular data format that is used for a variety of purposes. Here are some of the top use cases of JSON:
- Web APIs: JSON is often used to transmit data between web APIs and clients. This is because JSON is a lightweight and easy-to-parse format that can be easily transmitted over HTTP.
- Data storage: JSON can be used to store data in files or databases. This is because JSON is a human-readable format that can be easily understood and edited.
- Configuration files: JSON can be used to store configuration data for applications. This is because JSON is a flexible format that can be used to store a variety of data types.
- Log files: JSON can be used to store log data for applications. This is because JSON is a lightweight format that can be easily parsed and analyzed.
- Remote Procedure Calls (RPCs): JSON can be used to transmit data between RPC clients and servers. This is because JSON is a lightweight and easy-to-parse format that can be easily transmitted over HTTP.
What are the features of JSON?
JSON, or JavaScript Object Notation, is a lightweight data-interchange format. It is text-based and uses conventions that are familiar to programmers of the C family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
Here are some of the features of JSON:
- Lightweight: JSON is a lightweight format. This means that it is easy to read and write, and it takes up very little space.
- Human-readable: JSON is a human-readable format. This means that it can be easily understood by humans, even if they are not familiar with programming languages.
- Machine-readable: JSON is also a machine-readable format. This means that it can be easily parsed and processed by machines.
- Standardized: JSON is a standardized format. This means that there is a well-defined specification for how JSON should be formatted. This makes it easy to exchange JSON data between different applications.
- Extensible: JSON is an extensible format. This means that new data types can be easily added to JSON.
- Portable: JSON is a portable format. This means that it can be used on different platforms and with different programming languages.
What is the workflow of JSON?
The workflow of JSON is a flexible and efficient way to store and manipulate data. It is a popular format for data exchange between applications and for data storage in web applications.
Here are some additional details about each step in the workflow:
- Creating JSON data. When creating JSON data manually, it is important to follow the JSON syntax rules. These rules specify how the key-value pairs are formatted and how the data is structured.
- Storing JSON data. There are a variety of ways to store JSON data. One common way is to store it in a file. JSON files are typically text files with the .json extension. Another way to store JSON data is in a database. There are a number of databases that support JSON data, such as MongoDB and MySQL.
- Manipulating JSON data. There are a number of tools and programming languages that can be used to manipulate JSON data. One popular tool is the JSONLint website. This website can be used to validate JSON data and to identify any errors in the syntax. Another popular tool is the JSONPath library. This library can be used to search, sort, and filter JSON data.
- Serializing and deserializing JSON data. When JSON data is serialized, it is converted into a binary format for efficient storage and transmission. This process is called serialization. When JSON data is deserialized, it is converted back into its original format. This process is called deserialization.
How JSON Works & Architecture?
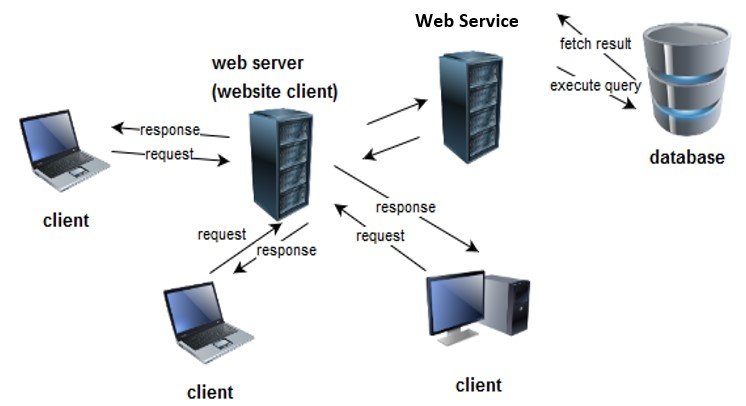
JSON (JavaScript Object Notation) is a text-based data interchange format that is easy for both humans and machines to understand. It’s not a program or software with a specific architecture; rather, it’s a standardized way of representing structured data. JSON’s architecture involves its syntax, data types, and how it is used in various contexts.
Let’s delve into how JSON works and its architecture:
JSON Syntax:
- JSON is based on a simple syntax that resembles the syntax of JavaScript object literals. It consists of the following components:
- Objects: Enclosed in curly braces {}, objects consist of key-value pairs, where keys are strings enclosed in double quotes, followed by a colon, and then the associated value.
- Arrays: Enclosed in square brackets [], arrays are ordered lists of values, which can include strings, numbers, objects, arrays, booleans, or null.
- Key-Value Pairs: Objects are made up of key-value pairs, where the key is a string and the value can be any JSON data type.
- Data Types: JSON supports several basic data types: strings, numbers (integers and floats), booleans (true or false), objects, arrays, and null.
How to Install and Configure JSON?
JSON (JavaScript Object Notation) is not a software or tool that needs to be installed or configured. It is a data interchange format that is built into most programming languages and environments. You can work with JSON data directly in your code without any installation or configuration steps.
Here’s how you can use JSON in different programming languages:
1. JavaScript:
JSON is native to JavaScript. You can create and parse JSON objects directly without any additional installation.
Example:
javascript
// Creating a JSON object in JavaScript
const person = {
name: "John Doe",
age: 30,
isStudent: false
};
2. Python:
Python includes a built-in module called json that provides functions for working with JSON data.
Example:
python
import json
Creating and using JSON data in Python
person = {
"name": "John Doe",
"age": 30,
"isStudent": False
}
json_data = json.dumps(person) # Serialize to JSON string
parsed_data = json.loads(json_data) # Deserialize JSON string to Python data
3. Java:
In Java, you can use libraries like Google’s Gson or Jackson to work with JSON data.
Example using Gson:
java
Copy code
import com.google.gson.Gson;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
// Creating and using JSON data in Java
String json = gson.toJson(new Person("John Doe", 30, false));
Person person = gson.fromJson(json, Person.class);
}
}
4. C#:
In C#, you can work with JSON using libraries like Newtonsoft.Json (Json.NET) or the built-in System.Text.Json namespace.
Example using Json.NET:
csharp
using Newtonsoft.Json;
class Program
{
static void Main(string[] args)
{
// Creating and using JSON data in C#
Person person = new Person("John Doe", 30, false);
string json = JsonConvert.SerializeObject(person);
Person deserializedPerson = JsonConvert.DeserializeObject(json);
}
}
[…] Fundamental Tutorials of JSON […]