Fundamental Tutorials of jQuery
What is jQuery?
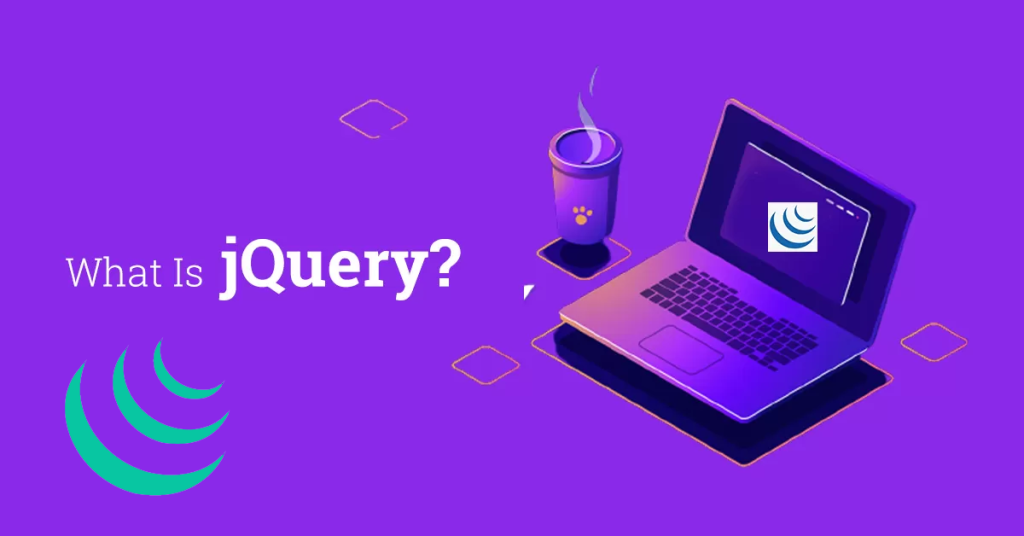
jQuery is a fast, lightweight, and feature-rich JavaScript library that simplifies various aspects of client-side web development. It provides a set of functions and utilities that make it easier to interact with HTML documents, manipulate the Document Object Model (DOM), handle events, create animations, make AJAX requests, and perform other common tasks.
jQuery is a popular choice for JavaScript because it is:
- Fast: jQuery is a lightweight library that does not add a lot of overhead to your website.
- Easy to learn: jQuery’s syntax is easy to learn, even for beginners.
- Extensive documentation: jQuery has extensive documentation and tutorials available online.
- Widely supported: jQuery is supported by all major browsers.
What are the top use cases of jQuery?
Here are some of the top use cases of jQuery:
- Creating interactive web pages: jQuery can be used to create interactive web pages with features such as dropdown menus, tabbed panels, and lightboxes.
- Developing AJAX applications: jQuery can be used to develop AJAX applications that allow users to interact with web pages without having to reload the page.
- Creating cross-browser-compatible web pages: jQuery is cross-browser compatible, which means that it can be used to create web pages that work in all major browsers.
- Simplifying JavaScript code: jQuery can be used to simplify JavaScript code by providing a number of shortcuts for common tasks.
- Making JavaScript code more readable: jQuery code is typically more readable than plain JavaScript code.
What are the features of jQuery?
jQuery is a JavaScript library that provides a number of features for simplifying HTML DOM tree traversal and manipulation, as well as event handling, CSS animation, and Ajax.
Here are some of the features of jQuery:
- DOM manipulation: jQuery provides a number of methods for manipulating the HTML DOM, such as selecting elements, adding and removing elements, and changing the content of elements.
- Event handling: jQuery provides a number of methods for handling events, such as clicks, mouse movements, and keyboard input.
- CSS animation: jQuery provides a number of methods for animating CSS properties, such as the position, size, and color of elements.
- AJAX: jQuery provides a number of methods for making AJAX requests, which are used to send and receive data from a web server without reloading the page.
- Plugins: There are a large number of jQuery plugins available that extend the functionality of jQuery.
What is the workflow of jQuery?
The workflow of jQuery is as follows:
Include the jQuery library in your HTML page. This can be done by adding the following code to your section:
HTML
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
- Write your jQuery code. jQuery code is typically written in the section of your HTML page.
- Call jQuery methods. jQuery methods are used to perform tasks such as selecting elements, adding and removing elements, and changing the content of elements.
- Bind events. Events are used to respond to user input. For example, you could bind an event to a button so that the button changes color when the user hovers over it.
- Animate CSS properties. jQuery can be used to animate CSS properties, such as the position, size, and color of elements.
- Make AJAX requests. AJAX requests are used to send and receive data from a web server without reloading the page.
- Use plugins. There are a large number of jQuery plugins available that extend the functionality of jQuery.
How jQuery Works & Architecture?
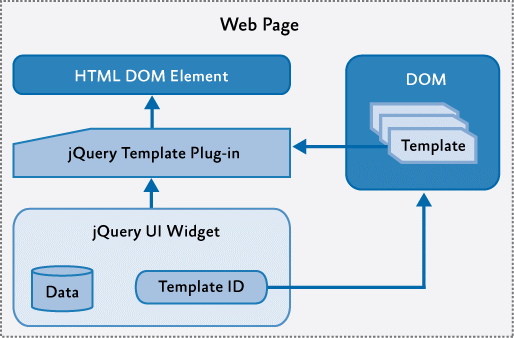
jQuery works by simplifying the Document Object Model (DOM) and providing a number of shortcuts for common tasks. The DOM is a tree-like structure that represents the HTML elements on a web page. jQuery provides a number of methods for selecting, adding, removing, and modifying elements in the DOM.
jQuery also provides a number of methods for handling events, such as clicks, mouse movements, and keyboard input. Events are used to respond to user input. For example, you could use jQuery to create a button that changes color when the user hovers over it.
jQuery also provides a number of methods for animating CSS properties, such as the position, size, and color of elements. CSS animations can be used to create dynamic and interactive web pages.
Finally, jQuery provides a number of methods for making AJAX requests. AJAX requests are used to send and receive data from a web server without reloading the page. This can be used to create web pages that are more responsive and interactive.
The architecture of jQuery is relatively simple. The jQuery library is a single JavaScript file that contains all of the jQuery methods. The jQuery methods are organized into namespaces, such as the $.fn namespace for DOM manipulation and the $.event namespace for event handling.
When you use a jQuery method, the jQuery library first parses the method name and then executes the corresponding code. The jQuery code is typically written in a concise and readable style.
Overall, jQuery is a powerful and versatile JavaScript library that can be used to simplify the DOM, handle events, animate CSS properties, and make AJAX requests. The jQuery library is well-documented and supported, and there are a large number of jQuery plugins available that extend the functionality of jQuery.
Here are some of the key concepts of jQuery architecture:
- The $ object: The $ object is the global object in jQuery. It is used to access all of the jQuery methods.
- The $.fn namespace: The $.fn namespace contains all of the jQuery methods for DOM manipulation.
- The $.event namespace: The $.event namespace contains all of the jQuery methods for event handling.
- The jQuery core: The jQuery core is the core library of jQuery. It contains the basic functionality of jQuery, such as DOM manipulation, event handling, and CSS animation.
- The jQuery plugins: The jQuery plugins are extensions to the jQuery core. They add additional functionality to jQuery, such as drag-and-drop, tabbed panels, and lightboxes.
How to Install and Configure jQuery?
There are two ways to install and configure jQuery:
1. Download the jQuery library and include it in your HTML page. This is the traditional way to install jQuery. You can download the jQuery library from the jQuery website. Once you have downloaded the library, you need to include it in your HTML page. This can be done by adding the following code to your section:
HTML
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
2. Use a CDN to host the jQuery library. A CDN is a Content Delivery Network. It is a network of servers that deliver content, such as JavaScript libraries, to users. There are a number of CDNs that host the jQuery library. You can use a CDN to host the jQuery library by adding the following code to your section:
HTML
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Once you have installed the jQuery library, you need to configure it. This can be done by adding the following code to your HTML page:
HTML
<script>
$(document).ready(function() {
// Your jQuery code goes here
});
</script>
The (document).ready()\ function is used to execute the jQuery code when the document is ready. The (document)
object is the global object in jQuery. It is used to access all of the jQuery methods.
Once you have configured jQuery, you can start using it to create dynamic and interactive web pages.
Here are some of the benefits of using a CDN to host the jQuery library:
- Performance: CDNs are designed to deliver content quickly and efficiently. This can improve the performance of your web pages.
- Scalability: CDNs can scale to handle large amounts of traffic. This means that your web pages will be able to handle even the most demanding traffic.
- Security: CDNs are typically hosted in secure locations. This means that your web pages will be protected from attacks.