Fundamental Tutorials of Dataframe
What is Dataframe?
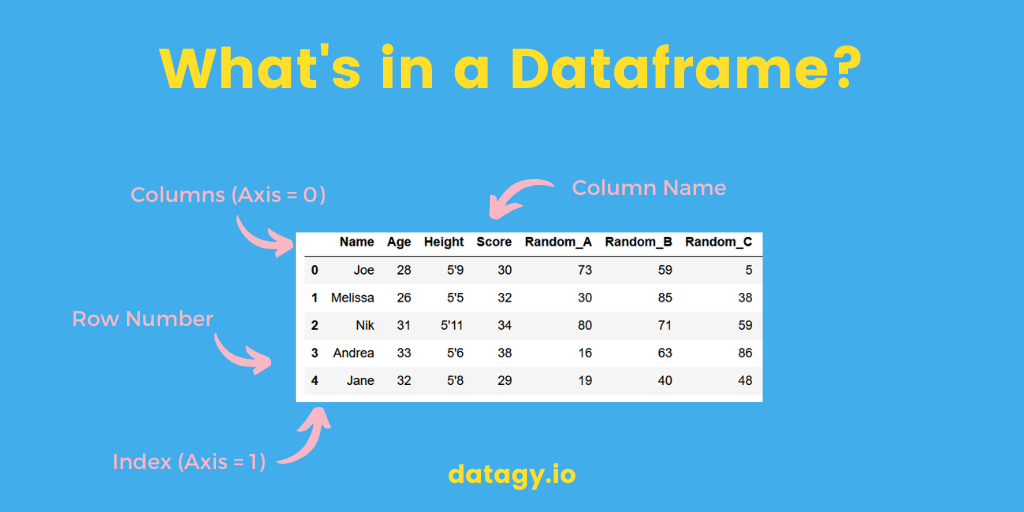
A DataFrame is a data structure in Python that is used to store and manipulate data. It is a two-dimensional table that is organized into rows and columns. Each row represents a single record, and each column represents a single field of data. DataFrames are similar to spreadsheets, but they are more powerful and flexible. DataFrames can be used to store data of any type, including strings, numbers, and dates. They can also be used to perform a variety of data manipulation operations, such as filtering, sorting, and aggregating data.
DataFrames are a popular data structure in Python for a variety of reasons. They are:
- Efficient: DataFrames are very efficient at storing and manipulating data. They can be used to store large amounts of data, and they can be used to perform complex data manipulation operations without slowing down your code.
- Flexible: DataFrames can be used to store data of any type. This makes them a versatile data structure that can be used for a variety of tasks.
- Easy to use: DataFrames are easy to use, even for beginners. They have a simple and intuitive API that makes it easy to learn how to use them.
What are the top use cases of Dataframe?
DataFrames are structured data abstraction in programming languages like Python (Pandas library) and R (data.frame) that provide a tabular and flexible way to manipulate and analyze data. They are particularly useful for data preprocessing, analysis, and transformation tasks.
Here are some of the top use cases of DataFrames:
- Data analysis: DataFrames are a powerful tool for data analysis. They can be used to clean, transform, and analyze data to extract insights.
- Machine learning: DataFrames are a popular data structure for machine learning. They can be used to store training data, to train machine learning models, and to evaluate machine learning models.
- Data visualization: DataFrames can be used to create data visualizations. This can be helpful for communicating data insights to others.
- Web scraping: DataFrames can be used to scrape data from websites. This can be helpful for collecting data that is not available in a structured format.
- Data integration: DataFrames can be used to integrate data from different sources. This can be helpful for creating a single view of data.
What are the features of a dataframe?
DataFrames are powerful data structures used for organizing and manipulating tabular data in programming languages like Python (Pandas library) and R (data.frame). They offer a range of features that make them highly versatile and suitable for various data analysis tasks.
Here are some of the features of DataFrames:
- Two-dimensional data structure: DataFrames are two-dimensional data structures, which means that they are organized into rows and columns. This makes them similar to spreadsheets.
- Labeled axes: The rows and columns of a DataFrame are labeled, which makes it easy to identify the data that is stored in each row and column.
- Heterogeneous data: DataFrames can store data of any type, including strings, numbers, and dates. This makes them a versatile data structure that can be used for a variety of tasks.
- Efficient data manipulation: DataFrames are very efficient at storing and manipulating data. They can be used to store large amounts of data, and they can be used to perform complex data manipulation operations without slowing down your code.
- Easy to use: DataFrames are easy to use, even for beginners. They have a simple and intuitive API that makes it easy to learn how to use them.
What is the workflow of Dataframe?
The workflow of DataFrame can vary depending on the specific task that you are trying to accomplish. However, there are a few common steps that you might follow:
- Load the DataFrame: The first step is to load the DataFrame from a file or database. You can use the read_csv() method to load a DataFrame from a CSV file, or the read_sql() method to load a DataFrame from a database.
- Clean the DataFrame: Once the DataFrame is loaded, you might need to clean it. This could involve removing duplicate rows, filling in missing values, or converting data types.
- Transform the DataFrame: Once the DataFrame is clean, you might need to transform it. This could involve pivoting, aggregating, or joining DataFrames.
- Analyze the DataFrame: Once the DataFrame is transformed, you can analyze it. This could involve performing statistical tests, creating visualizations, or building machine learning models.
- Save the DataFrame: Once you are finished with the DataFrame, you might want to save it to a file or database. You can use the to_csv() method to save a DataFrame to a CSV file, or the to_sql() method to save a DataFrame to a database.
How Dataframe Works & Architecture?
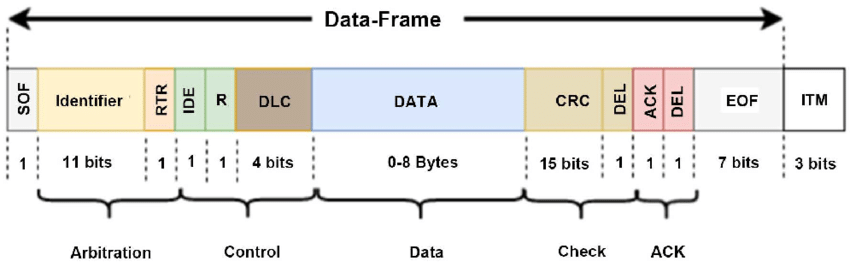
DataFrames are a powerful data structure that can be used to store, manipulate, and analyze data. They are built on top of NumPy arrays, which are a fast and efficient way to store and manipulate numerical data. DataFrames add a layer of abstraction on top of NumPy arrays, making it easier to work with data in a tabular format. DataFrames are organized into rows and columns, just like a spreadsheet. Each row represents a single data record, and each column represents a single field of data. The data in a DataFrame can be of any type, including strings, numbers, and dates.
DataFrames are a popular data structure for a variety of tasks, including:
- Data analysis: DataFrames can be used to clean, transform, and analyze data to extract insights.
- Machine learning: DataFrames are a popular data structure for machine learning. They can be used to store training data, to train machine learning models, and to evaluate machine learning models.
- Data visualization: DataFrames can be used to create data visualizations. This can be helpful for communicating data insights to others.
- Web scraping: DataFrames can be used to scrape data from websites. This can be helpful for collecting data that is not available in a structured format.
- Data integration: DataFrames can be used to integrate data from different sources. This can be helpful for creating a single view of data.
How to Install and Configure Dataframe?
DataFrames are not standalone software or library to be installed and configured independently. Instead, DataFrames are data structures provided by specific libraries in programming languages like Python and R. One of the most widely used libraries for DataFrames is Pandas in Python.
Here’s how you can install and use Pandas to work with DataFrames:
1. Install Pandas
To install Pandas, you can use the following command in your Python environment (such as Anaconda or a virtual environment):
pip install pandas
2. Import Pandas:
In your Python script or Jupyter notebook, import the Pandas library:
import pandas as pd
3. Create a DataFrame:
You can create a DataFrame using various methods, such as from dictionaries, lists, NumPy arrays, or reading data from files (CSV, Excel, etc.). Here’s an example using a dictionary:
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 22],
'City': ['New York', 'San Francisco', 'Los Angeles']}
df = pd.DataFrame(data)
4. Data Manipulation and Analysis:
Once you have a DataFrame, you can perform various data manipulation and analysis tasks using Pandas methods. Here are a few examples:
- Display the first few rows: df.head()
- Get summary statistics: df.describe()
- Filter rows: df[df[‘Age’] > 25]
- Group and aggregate data: df.groupby(‘City’)[‘Age’].mean()
- Create new columns: df[‘Status’] = ‘Active’
5. Data Visualization:
You can also visualize data using libraries like Matplotlib or Seaborn. Here’s an example using Matplotlib:
import matplotlib.pyplot as plt
df.plot(kind='bar', x='Name', y='Age')
plt.xlabel('Name')
plt.ylabel('Age')
plt.title('Age Distribution')
plt.show()
Keep in mind that the above steps are specifically for installing and using Pandas to work with DataFrames in Python. If you’re using R, you can use the built-in data.frame structure for DataFrames.
[…] Fundamental Tutorials of Dataframe […]