Fundamental Tutorials of Array
What is Array?
An array is a fundamental data structure in programming that allows you to store and organize multiple values of the same type under a single variable name. Each value in an array is called an element, and each element is associated with an index that represents its position within the array. Arrays are commonly used to store collections of related data, such as a list of numbers, strings, objects, or any other data type.
Arrays are one of the most common data structures in computer science. They are used in a wide variety of applications, including:
- Storing lists of data
- Sorting data
- Searching data
- Implementing mathematical operations
Arrays are one of the most common data structures in computer science, and they are used in a wide variety of applications.
What are the top use cases of Array?
Here are some of the top use cases of arrays:
- Storing lists of data. Arrays are a natural way to store lists of data. For example, you could use an array to store a list of students’ names, a list of products in a store, or a list of websites that you have visited.
- Sorting data. Arrays can be sorted in ascending or descending order using a variety of sorting algorithms. This makes it easy to find the largest or smallest element in an array or to find all of the elements that are greater than or less than a certain value.
- Searching data. Arrays can be searched for specific elements using algorithms such as linear search and binary search. This makes it possible to find an element in an array quickly, even if the array contains a large number of elements.
- Implementing mathematical operations. Arrays can be used to implement a variety of mathematical operations, such as finding the sum, average, or median of a set of numbers.
- Implementing data structures. Arrays can be used to implement a variety of data structures, such as stacks, queues, and linked lists.
What are features of Array?
Arrays have a number of features that make them a powerful and versatile data structure.
These features include:
- Homogeneity: Arrays can only store elements of the same data type. This means that all of the elements in an array must be integers, or all of the elements must be strings, and so on.
- Contiguous memory: The elements in an array are stored in contiguous memory locations. This means that they are stored next to each other in memory, which makes it easy to access the elements in an array by their index.
- Indexing: Each element in an array has a unique index. An index is a number that identifies the element’s position in the array. For example, the first element in an array has an index of 0, the second element has an index of 1, and so on.
- Size: The size of an array is the number of elements that it can store. The size of an array is fixed, so it cannot be changed after the array is created.
- Iteration: Arrays can be iterated by using a for loop. This allows you to access each element in the array one at a time.
- Slicing: Arrays can be sliced to create a new array that contains a subset of the original array’s elements. This is useful for extracting specific elements from an array or for creating a new array that contains a subset of the original array’s elements.
What is the workflow of Array?
The workflow of an array is as follows:
- Declaration: The first step is to declare the array. This is done by specifying the data type of the elements in the array, as well as the size of the array.
- Initialization: The next step is to initialize the array. This can be done by assigning values to the elements in the array, or by leaving the elements uninitialized.
- Accessing elements: Once the array is declared and initialized, you can access the elements in the array by their index. An index is a number that identifies the element’s position in the array.
- Modifying elements: You can also modify the elements in the array. This can be done by assigning new values to the elements, or by deleting elements from the array.
- Iterating through the array: You can iterate through the array using a for loop. This allows you to access each element in the array one at a time.
- Slicing the array: You can slice the array to create a new array that contains a subset of the original array’s elements. This is useful for extracting specific elements from an array or for creating a new array that contains a subset of the original array’s elements.
- Deleting the array: When you are finished with the array, you can delete it. This frees up the memory that was used by the array.
How Array Works & Architecture?
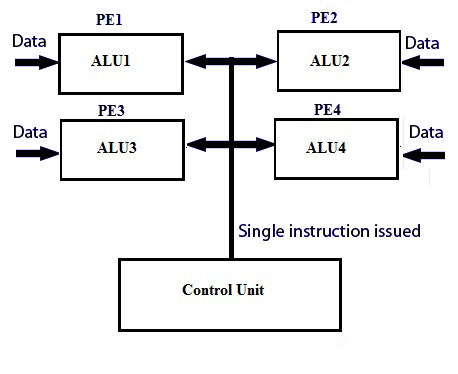
When an array is created, the array header is allocated in memory. The array elements are then allocated in memory, starting at the address that is specified in the array header. The size of the array is determined by the number of elements that are allocated in memory. The elements in an array can be accessed by their index. The index is a number that identifies the element’s position in the array. For example, the first element in an array has an index of 0, the second element has an index of 1, and so on.
The elements in an array can be modified by assigning new values to them. The elements in an array can also be deleted by freeing up the memory that they are using.
Here are some additional details about the architecture of an array:
- The array header is typically a fixed size, regardless of the size of the array.
- The array elements are typically stored in a contiguous block of memory.
- The size of the array is determined by the number of elements that are allocated in memory.
- The elements in an array can be accessed by their index.
- The elements in an array can be modified by assigning new values to them.
- The elements in an array can be deleted by freeing up the memory that they are using.
How to Install and Configure Array?
Arrays are fundamental data structures provided by programming languages, and they don’t require separate installation or configuration steps. They are built-in features that you can use directly in your code. Here’s how you can work with arrays in a few common programming languages:
1. JavaScript:
Arrays are an integral part of JavaScript and are available without any installation or configuration.
Example:
javascript
// Creating and using an array in JavaScript
const numbers = [1, 2, 3, 4, 5];
console.log(numbers[0]); // Outputs: 1
2. Python:
Arrays in Python are usually implemented using lists, which are built-in data structures.
Example:
python
# Creating and using a list (array) in Python
numbers = [1, 2, 3, 4, 5]
print(numbers[0]) # Outputs: 1
3. Java:
In Java, arrays are part of the language’s core features. No separate installation is needed.
Example:
Java
// Creating and using an array in Java
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(numbers[0]); // Outputs: 1
4. C++:
Arrays are supported in C++ and can be used without any extra installation.
Example:
cpp
// Creating and using an array in C++
int numbers[] = {1, 2, 3, 4, 5};
std::cout << numbers[0] << std::endl; // Outputs: 1
5. C# (C Sharp):
Arrays are a fundamental feature of C# and don’t require separate installation.
Example:
csharp
// Creating and using an array in C#
int[] numbers = {1, 2, 3, 4, 5};
Console.WriteLine(numbers[0]); // Outputs: 1
[…] Fundamental Tutorials of Array […]