Fundamental Tutorials of AJAX
What is AJAX?
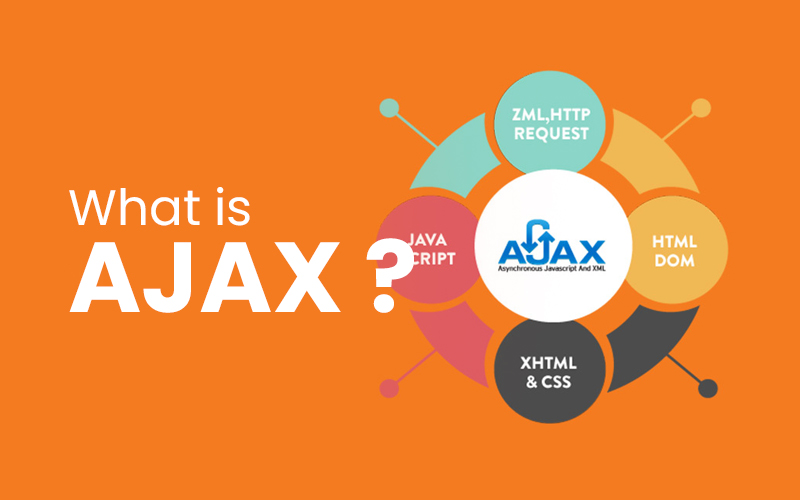
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that uses various web technologies on the client side to create asynchronous web applications. With AJAX, web applications can send and retrieve data from a server asynchronously without interfering with the display and behavior of the existing page. This means that web pages can be updated without having to reload the entire page, which can improve the user experience and make web applications more responsive.
AJAX is used in a variety of web applications, including:
- Email clients: AJAX is used to fetch new emails without having to reload the entire inbox.
- Social media platforms: AJAX is used to update news feeds and status updates without having to reload the entire page.
- Chat applications: AJAX is used to send and receive messages without having to reload the entire chat window.
- Online banking: AJAX is used to update account balances and transaction history without having to reload the entire banking page.
What are the top use cases of AJAX?
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that allows web pages to be updated without having to reload the entire page. This can improve the user experience and make web applications more responsive.
Here are some of the top use cases of AJAX:
- Real-time updates: AJAX can be used to update web pages in real-time, without having to reload the entire page. This is useful for applications like stock tickers, news feeds, and chat applications.
- Form validation: AJAX can be used to validate forms on the client side before they are submitted to the server. This can help to prevent errors and improve the user experience.
- Dynamic content: AJAX can be used to add dynamic content to web pages, such as product recommendations, weather forecasts, and maps. This can make web pages more engaging and informative.
- Drag and drop: AJAX can be used to implement drag and drop functionality on web pages. This can be useful for applications like file uploading and image editing.
- Autocomplete: AJAX can be used to implement autocomplete functionality on web pages. This can save users time and effort when entering data.
What are the features of AJAX?
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that uses various web technologies on the client side to create asynchronous web applications. With AJAX, web applications can send and retrieve data from a server asynchronously without interfering with the display and behavior of the existing page.
Here are some of the features of AJAX:
- Asynchronous: AJAX requests are made asynchronously, which means that they do not block the main thread of the web browser. This allows web pages to be updated without having to reload the entire page.
- Data-driven: AJAX applications are data-driven, which means that they can be updated with new data without having to be rewritten. This makes AJAX applications more flexible and adaptable to changes in data.
- Cross-platform: AJAX applications are cross-platform, which means that they can be used on a variety of devices, including computers, tablets, and smartphones. This makes AJAX applications more accessible to a wider range of users.
- Extensible: AJAX is an extensible technology, which means that it can be used to create a variety of different web applications. This makes AJAX a versatile tool that can be used to solve a variety of problems.
What is the workflow of AJAX?
The workflow of AJAX is as follows:
- The user performs an action on the web page, such as clicking a button or entering text into a form.
- The JavaScript code on the web page creates an XMLHttpRequest object.
- The XMLHttpRequest object sends a request to the server.
- The server processes the request and sends a response back to the client.
- The XMLHttpRequest object receives the response from the server.
- The JavaScript code on the web page parses the response and updates the web page.
How AJAX Works & Architecture?
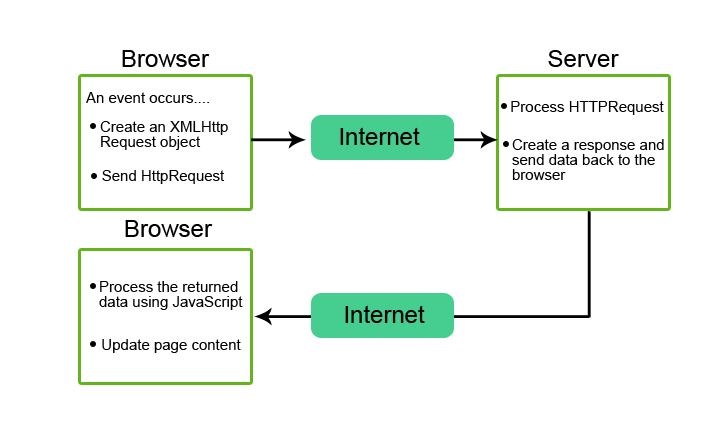
AJAX stands for Asynchronous JavaScript and XML. It is a set of web development techniques that uses various web technologies on the client side to create asynchronous web applications. With AJAX, web applications can send and retrieve data from a server asynchronously without interfering with the display and behavior of the existing page.
Here is the architecture of AJAX:
- XMLHttpRequest object: The XMLHttpRequest object is a JavaScript object that allows the web page to communicate with the server asynchronously.
- JavaScript code: The JavaScript code on the web page is responsible for creating the XMLHttpRequest object, sending the request to the server, receiving the response from the server, and parsing the response.
- Server-side code: The server-side code is responsible for processing the request from the client and sending the response back to the client.
How to Install and Configure AJAX?
Unlike programming languages or frameworks, AJAX (Asynchronous JavaScript and XML) is not something that you install or configure separately. Instead, AJAX is a technique or approach that involves using JavaScript to make asynchronous requests to a server and update parts of a web page without requiring a full page reload. It is an integral part of modern web development, and you can implement it directly within your HTML and JavaScript code.
Here’s a high-level overview of how to use AJAX in your web application:
1. Include JavaScript Library:
You don’t need to install anything specific to use AJAX, but JavaScript is required. Most modern browsers support the built-in XMLHttpRequest object for making AJAX requests. Alternatively, you can use libraries like jQuery, Axios, or the fetch API to simplify the process of making AJAX requests.
2. Write JavaScript Code:
You’ll need to write JavaScript code that handles the AJAX request and response. This code typically involves creating an instance of XMLHttpRequest or using a library-specific function to send a request to the server.
Here’s a basic example using the XMLHttpRequest object:
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
var responseData = xhr.responseText;
// Update the DOM with the retrieved data
}
};
xhr.send();
- Handle the Response:
In the example above, the onreadystatechange event handler is used to monitor the status of the request. Once the response is received (readyState === 4) and the status code indicates success (status === 200), you can process the response data and update the DOM as needed.
2. Update the DOM:
After receiving the response, you can use JavaScript to update specific elements on the page. This could involve adding new content, modifying existing content, or performing other actions based on the data from the server.
It’s important to note that the configuration and usage of AJAX depend on your specific application and requirements. Additionally, you may need to handle issues like cross-origin requests, error handling, and security considerations.