How to decrease your Gradle build time by 65%?
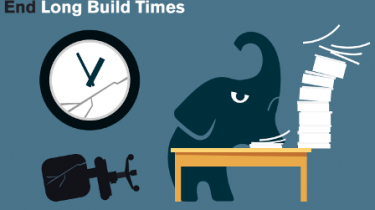
Source:- kevalpatel2106.wordpress.com
Gradle is very powerful build system. It handles the very complex process of building .dx files from java source code, merging all the resources and assets into the application package (.apk) and signing the application.
But gradle isn’t perfect. It is comparatively slower than other build systems out there.
Why should you care about build time of your project?
For a long time, I was OKAY with my build timings. I thought I can live with this. I used to do other stuff like surfing the internet, making coffee and even taking my lunch while gradle builds my project.
Now, let’s say you work on the above I/O’16 application project for 6 hours a day and run gradle build to install debug apk file on your test device for almost 15 times, you will spend 35 minutes a day and almost 3 hours/week(almost 10% of total time) just sitting idle and watching gradle build running.
That’s why it is very important that you optimize your build timing. The less time you spend watching gradle build your project, the more you can be productive. After all, time is precious.
I applied some modifications in my build process to decrease the build time and here are a few tips for you that will help you to decrease your build timings.
Here for example purpose, we will use Google I/O application as the sample project. We are going to run all the benchmarks on that project. This project contains more than 28 dependencies and 41538 method references. Also, the source code of the application is openly available on GitHub.
Build without any modifications:
Let’s measure the time to build and debug app from the project with the current configuration using below command.
./gradlew android:assembleDebug --profile
— profile will tell gradle to measure the time taken to execute each task and dump that data into HTML file. You can find that report under /projectDir/build/reports/profile directory.
- As you can see it takes 2 minutes and 43 seconds to build the debug apk from the source on my machine. Keep this figure in your mind.
These benchmark timings may vary on your machine depending on your system configuration. Here I am going to use my 13″ Macbook Pro 2016 with touch barto run all these tasks.
Now, let’s try to decrease this time.
Gradle Build Cycle:
Gradle has three distinct phases in build life cycle:
- Initialisation: In this stage of the build life cycle, gradle picks the project and decides what things to build.
- Configuration: Here, gradle will evaluate your build.gradle script, configure all the plugins and evaluate the task graph.
- Execution: In this phase gradle actually runs all the task those were evaluated in previous phase to get the work done and build the application.
As you can see that out of all three phases, only execution phase performs all the operations. That means that first two phases are just overhead to the gradle build process. We don’t care about them. But, sadly whenever you do anything with gradle this two phases will always get executed.
So, how can we measure the time taken to execute first two phases? Luckily, gradle provides — dry-run command, that tells gradle to evaluate the project but don’t run any task. Thus execution phase won’t get executed. So, run this command in your terminal:
./gradlew android:assembleDebug --dry-run --profile
Here is the time taken by first two phase on over Google I/O project.
You can see in above statistics that gradle passed almost 7.8 seconds just to initialise and configure the project. That is completely waste of time for us.
Let’s see if we can decrease the time taken to initialise and configure the project.
Configure on demand:
Google I/O app has two components/modules:
- Android : That contains the source code related to the andorid application and
- Server : This contains code related to backend server.
Now, if you are building an android application, you don’t want to configure server component. Right? But previous statistic shows that gradle passed almost a second to just configure server component.
Gradle provides — configure -on-demand flag, that will tell gradle to only build the projects that it really needs to build. Let’s run this command.
./gradlew android:assembleDebug --dry-run --profile --configure-on-demand
As you can see, if we use — configure-on-demand, gradle will skip configuring the server module and it will decrease the build time by almost a second. I know this is not a huge gain, but small drops can fill the whole lake!!!
Time decreased : 1 second (6% win).
How you can enable configure-on-demand in android studio?
You can enable — configure-on-demand for every build you can add org.gradle.configureondemand=true in your gradle.properties file or in your android studio, navigate to Preferences > Build, Execution, Deployment > Compiler and check configure on demand option.
Enable daemon:
Gradle has a very good feature called Gradle Daemon. Daemon keeps the instance of the gradle up and running in the background even after your build finishes. This will remove the time required to initialize the gradle and decrease your build timing significantly.
You won’t be able to see the time difference in your first build as gradle has to initialize and start the daemon, but build times will decrease in subsequent builds as gradle daemon is already initialised.
./gradlew android:assembleDebug --dry-run --profile --configure-on-demand --daemon
In our case, the build time is decreased by about 4.5 seconds. Hurray!!!
Time decreased by : 4.5 seconds (71% win)
How you can enable daemon in android studio?
If you are using the gradle version 3.0 or above, the gradle daemon is by default enabled. But, if you are running on older versions of gradle then you can enable it for every build by adding org.gradle.daemon=true in your gradle.properties file.
Always keep your gradle up-to-date:
Gradle itself got faster with each new releases. There are many tweaks and performance improvements in the latest versions of gradle. Also, if you are running on gradle 2.4 or above, gradle will also cache build artifacts and that will improve the build time significantly.
So, always make sure that you are using the latest version of the gradle. You can go to /gradle/wrapper/gradle-wrapper.properties and upgrade gradle version by changing distributionUrl.
While writing this article, the gradle the latest gradle version is 3.3. Here is the updated gradle-wrapper.properties file for I/O application.
./gradlew android:assembleDebug --dry-run --profile --configure-on-demand --daemon
Time decreased by : 0.7 seconds (82% win ?)
So far we achived almost 82% gain in initialise and configuration timings. Now, let’s try to decrease the time required in execution phase.
Increase the heap size:
Since android studio 2.0, gradle uses dex in the process to decrease the build timings for the project.
“Dex in process” is a process that allows to run multiple dex processes to run within the single VM that is also shared with the gradle.
Generally, while building the applications, multiple dx processes runs on different VM instances.
But starting from the Android Studio 2.0, all these dx processes runs in the single VM and that VM is also shared with the gradle.(You need to have build tool version 23.0.2 or above to enable dex in the process.)
This decreases the build time significantly as all the dex process runs on the same VM instances. But this requires larger memory to accommodate all the dex processes and gradle. That means you need to increase the heap size required by the gradle daemon. By default, the heap size for the daemon is about 1GB. You have to increase the heap size by adding below line in your gradle.properties.
org.gradle.jvmargs=-Xmx3072m -XX:MaxPermSize=512m -XX:+HeapDumpOnOutOfMemoryError -Dfile.encoding=UTF-8
The required heap size varies from machine to machine. For my machine, I found that 3GB heap size is perfect. Any more increase in the heap size doesn’t affect the build timing significantly.
Now, let’s run full clean build using the below command:
./gradlew android:assembleDebug --profile --configure-on-demand --daemon
Time decrease by : 1 min 43 sec (63% win) — From 2 min 43 sec to 1 min
Modularise and Parallelise build:
Allow gradle to build your project in parallel. If you have multiple modules in you project, then by enabling this, gradle can run build operations for independent modules parallelly. This may decrease the build time significantly for very complex and multi-module projects.
You can enable parallelism by adding org.gradle.parallel=true in your gradle.properties file.
As our project doesn’t have multiple modules, building parallel won’t make much difference.
Time decrease by : 1 min 45 sec (64.5% win)
Other small things to remember:
– Avoid heavy computations, like increasing the version code from git commits count or downloading some files from url while building the project. Gradle will spend time to perform those computations or network operations and that will afftect build timings.
– Don’t use dynamic dependency like,
compile 'com.android.support:appcompat-v7:23.0.+'
If you are using the dynamic dependencies, gradle will go online and check if there is any newer version for the library is available or not? (Most of the time, it is just waste of the precious build time). Instead, use the fixed dependencies and update them manually time to time.
compile 'com.android.support:appcompat-v7:23.0.2'
TL;DR:
The long story short, add below lines to your gradle.properties file and you will get almost 65% decrease in your build time.
<gist>2fbf175066a0cadbb3af1008954ed077</gist>